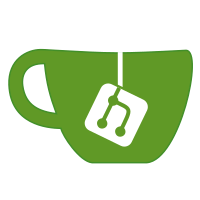 Terminal: change command-line parser (#2247)
Change the underlying command line handling:
- switch to a custom parser, inspired by redis / sds
- update terminalRegisterCommand signature, pass only bare minimum
- clean-up `help` & `commands`. update settings `set`, `get` and `del`
- allow our custom test suite to run command-line tests
- clean-up Stream IO to allow us to print large things into debug stream (for example, `eeprom.dump`)
- send parsing errors to the debug log
As a proof of concept, introduce `TERMINAL_MQTT_SUPPORT` and `TERMINAL_WEB_API_SUPPORT`
- MQTT subscribes to the `<root>/cmd/set` and sends response to the `<root>/cmd`. We can't output too much, as we don't have any large-send API.
- Web API listens to the `/api/cmd?apikey=...&line=...` (or PUT, params inside the body). This one is intended as a possible replacement of the `API_SUPPORT`. Internals introduce a 'task' around the AsyncWebServerRequest object that will simulate what WiFiClient does and push data into it continuously, switching between CONT and SYS.
Both are experimental. We only accept a single command and not every command is updated to use Print `ctx.output` object. We are also somewhat limited by the Print / Stream overall, perhaps I am overestimating the usefulness of Arduino compatibility to such an extent :)
Web API handler can also sometimes show only part of the result, whenever the command tries to yield() by itself waiting for something. Perhaps we would need to create a custom request handler for that specific use-case. 4 years ago |
|
- /*
-
- Part of the TERMINAL MODULE
-
- Copyright (C) 2016-2019 by Xose Pérez <xose dot perez at gmail dot com>
- Copyright (C) 2020 by Maxim Prokhorov <prokhorov dot max at outlook dot com>
-
- */
-
- #pragma once
-
- #include <Arduino.h>
- #include <vector>
-
- namespace terminal {
- namespace parsing {
-
- // Generic command line parser
- // - split each arg from the input line and put them into the argv array
- // - argc is expected to be equal to the argv
- struct CommandLine {
- std::vector<String> argv;
- size_t argc;
- };
-
- CommandLine parse_commandline(const char *line);
-
- // Fowler–Noll–Vo hash function to hash command strings that treats input as lowercase
- // ref: https://en.wikipedia.org/wiki/Fowler%E2%80%93Noll%E2%80%93Vo_hash_function
- //
- // TODO: afaik, unordered_map should handle collisions (however rare they are in our case)
- // if not, we can always roll static commands allocation and just match strings
- // with LowercaseEquals (which is not that much slower)
- template <typename T>
- struct LowercaseFnv1Hash {
- size_t operator()(const T& str) const;
- };
-
- template <typename T>
- struct LowercaseEquals {
- bool operator()(const T& lhs, const T& rhs) const;
- };
-
- }
- }
|