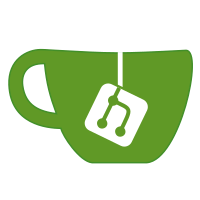 Button pin provider & runtime settings (#2162)
* wip
* cleanup buttons-through-serial code, remove hw mention from button module
* remove wip
* implement mqtt settings
* fixup struct members, dual no longer allocates debouncer
* add missing debounceevent lib
* fix missing event_handler, update names
* fix namespace
* drop lib
* fix int<->bool comparison
* Move gpio16 handling from DigitalPin to EventHandler
* Cleanup debounceevent headers
* Don't expect system headers to be included
* re 70b54c489f - no allocation, for real
* Adjust settings names
* dont retain by default
* unused
* typo
* Fix length type (ref 6017ad9474c5ea2f77fbca5f3c19b6cef058b2e9)
* Move pin class outside of debounce lib, lowercase ns
* move event handling inside of button_t
* refactor config. ..._MODE -> _CONFIG, ..._MODE_... -> ..._ACTION_...
* fix test
* naming
* move indexed value to header
* refactor actions into direct opts
* fix webui, fix buttons not respecting old user setting
* change button config format from bitmask to a struct, adjust settings conversion
* proxy some more header defautls, fix web kv
* gpiopin
* adjust webui func to support every setting
* clarify single-return event->string
* fix dual setting
* fix dual packet condition, de-duplicate funcs
* fix bogus warning 4 years ago 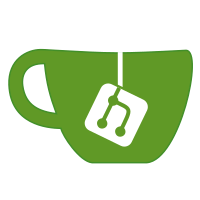 Terminal: change command-line parser (#2247)
Change the underlying command line handling:
- switch to a custom parser, inspired by redis / sds
- update terminalRegisterCommand signature, pass only bare minimum
- clean-up `help` & `commands`. update settings `set`, `get` and `del`
- allow our custom test suite to run command-line tests
- clean-up Stream IO to allow us to print large things into debug stream (for example, `eeprom.dump`)
- send parsing errors to the debug log
As a proof of concept, introduce `TERMINAL_MQTT_SUPPORT` and `TERMINAL_WEB_API_SUPPORT`
- MQTT subscribes to the `<root>/cmd/set` and sends response to the `<root>/cmd`. We can't output too much, as we don't have any large-send API.
- Web API listens to the `/api/cmd?apikey=...&line=...` (or PUT, params inside the body). This one is intended as a possible replacement of the `API_SUPPORT`. Internals introduce a 'task' around the AsyncWebServerRequest object that will simulate what WiFiClient does and push data into it continuously, switching between CONT and SYS.
Both are experimental. We only accept a single command and not every command is updated to use Print `ctx.output` object. We are also somewhat limited by the Print / Stream overall, perhaps I am overestimating the usefulness of Arduino compatibility to such an extent :)
Web API handler can also sometimes show only part of the result, whenever the command tries to yield() by itself waiting for something. Perhaps we would need to create a custom request handler for that specific use-case. 4 years ago 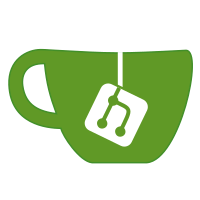 Terminal: change command-line parser (#2247)
Change the underlying command line handling:
- switch to a custom parser, inspired by redis / sds
- update terminalRegisterCommand signature, pass only bare minimum
- clean-up `help` & `commands`. update settings `set`, `get` and `del`
- allow our custom test suite to run command-line tests
- clean-up Stream IO to allow us to print large things into debug stream (for example, `eeprom.dump`)
- send parsing errors to the debug log
As a proof of concept, introduce `TERMINAL_MQTT_SUPPORT` and `TERMINAL_WEB_API_SUPPORT`
- MQTT subscribes to the `<root>/cmd/set` and sends response to the `<root>/cmd`. We can't output too much, as we don't have any large-send API.
- Web API listens to the `/api/cmd?apikey=...&line=...` (or PUT, params inside the body). This one is intended as a possible replacement of the `API_SUPPORT`. Internals introduce a 'task' around the AsyncWebServerRequest object that will simulate what WiFiClient does and push data into it continuously, switching between CONT and SYS.
Both are experimental. We only accept a single command and not every command is updated to use Print `ctx.output` object. We are also somewhat limited by the Print / Stream overall, perhaps I am overestimating the usefulness of Arduino compatibility to such an extent :)
Web API handler can also sometimes show only part of the result, whenever the command tries to yield() by itself waiting for something. Perhaps we would need to create a custom request handler for that specific use-case. 4 years ago |
|
- /*
-
- LightFox module
-
- Copyright (C) 2019 by Andrey F. Kupreychik <foxle@quickfox.ru>
-
- */
-
- #include "lightfox.h"
-
- #ifdef FOXEL_LIGHTFOX_DUAL
-
- // -----------------------------------------------------------------------------
- // DEFINITIONS
- // -----------------------------------------------------------------------------
-
- #define LIGHTFOX_CODE_START 0xA0
- #define LIGHTFOX_CODE_LEARN 0xF1
- #define LIGHTFOX_CODE_CLEAR 0xF2
- #define LIGHTFOX_CODE_STOP 0xA1
-
- // -----------------------------------------------------------------------------
- // PUBLIC
- // -----------------------------------------------------------------------------
-
- void lightfoxLearn() {
- Serial.write(LIGHTFOX_CODE_START);
- Serial.write(LIGHTFOX_CODE_LEARN);
- Serial.write(0x00);
- Serial.write(LIGHTFOX_CODE_STOP);
- Serial.println();
- Serial.flush();
- DEBUG_MSG_P(PSTR("[LIGHTFOX] Learn comman sent\n"));
- }
-
- void lightfoxClear() {
- Serial.write(LIGHTFOX_CODE_START);
- Serial.write(LIGHTFOX_CODE_CLEAR);
- Serial.write(0x00);
- Serial.write(LIGHTFOX_CODE_STOP);
- Serial.println();
- Serial.flush();
- DEBUG_MSG_P(PSTR("[LIGHTFOX] Clear comman sent\n"));
- }
-
- // -----------------------------------------------------------------------------
- // WEB
- // -----------------------------------------------------------------------------
-
- #if WEB_SUPPORT
-
- void _lightfoxWebSocketOnConnected(JsonObject& root) {
- root["lightfoxVisible"] = 1;
- uint8_t buttonsCount = _buttons.size();
- root["lightfoxRelayCount"] = relayCount();
- JsonArray& rfb = root.createNestedArray("lightfoxButtons");
- for (byte id=0; id<buttonsCount; id++) {
- JsonObject& node = rfb.createNestedObject();
- node["id"] = id;
- node["relay"] = getSetting({"btnRelay", id}, 0);
- }
- }
-
- void _lightfoxWebSocketOnAction(uint32_t client_id, const char * action, JsonObject& data) {
- if (strcmp(action, "lightfoxLearn") == 0) lightfoxLearn();
- if (strcmp(action, "lightfoxClear") == 0) lightfoxClear();
- }
-
- #endif
-
- // -----------------------------------------------------------------------------
- // TERMINAL
- // -----------------------------------------------------------------------------
-
- #if TERMINAL_SUPPORT
-
- void _lightfoxInitCommands() {
-
- terminalRegisterCommand(F("LIGHTFOX.LEARN"), [](const terminal::CommandContext&) {
- lightfoxLearn();
- DEBUG_MSG_P(PSTR("+OK\n"));
- });
-
- terminalRegisterCommand(F("LIGHTFOX.CLEAR"), [](const terminal::CommandContext&) {
- lightfoxClear();
- DEBUG_MSG_P(PSTR("+OK\n"));
- });
- }
-
- #endif
-
- // -----------------------------------------------------------------------------
- // SETUP & LOOP
- // -----------------------------------------------------------------------------
-
- void lightfoxSetup() {
-
- #if WEB_SUPPORT
- wsRegister()
- .onConnected(_lightfoxWebSocketOnConnected)
- .onAction(_lightfoxWebSocketOnAction);
- #endif
-
- #if TERMINAL_SUPPORT
- _lightfoxInitCommands();
- #endif
-
- }
-
- #endif
|