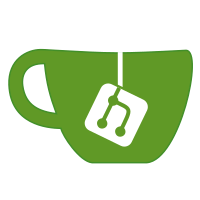 Terminal: change command-line parser (#2247)
Change the underlying command line handling:
- switch to a custom parser, inspired by redis / sds
- update terminalRegisterCommand signature, pass only bare minimum
- clean-up `help` & `commands`. update settings `set`, `get` and `del`
- allow our custom test suite to run command-line tests
- clean-up Stream IO to allow us to print large things into debug stream (for example, `eeprom.dump`)
- send parsing errors to the debug log
As a proof of concept, introduce `TERMINAL_MQTT_SUPPORT` and `TERMINAL_WEB_API_SUPPORT`
- MQTT subscribes to the `<root>/cmd/set` and sends response to the `<root>/cmd`. We can't output too much, as we don't have any large-send API.
- Web API listens to the `/api/cmd?apikey=...&line=...` (or PUT, params inside the body). This one is intended as a possible replacement of the `API_SUPPORT`. Internals introduce a 'task' around the AsyncWebServerRequest object that will simulate what WiFiClient does and push data into it continuously, switching between CONT and SYS.
Both are experimental. We only accept a single command and not every command is updated to use Print `ctx.output` object. We are also somewhat limited by the Print / Stream overall, perhaps I am overestimating the usefulness of Arduino compatibility to such an extent :)
Web API handler can also sometimes show only part of the result, whenever the command tries to yield() by itself waiting for something. Perhaps we would need to create a custom request handler for that specific use-case. 4 years ago |
|
- /*
-
- Part of the API MODULE
-
- Copyright (C) 2016-2019 by Xose Pérez <xose dot perez at gmail dot com>
- Copyright (C) 2020 by Maxim Prokhorov <prokhorov dot max at outlook dot com>
-
- */
-
- #include "espurna.h"
-
- #include "api.h"
-
- #include "ws.h"
- #include "web.h"
-
- // -----------------------------------------------------------------------------
-
- #if WEB_SUPPORT
-
- namespace {
-
- bool _apiWebSocketOnKeyCheck(const char * key, JsonVariant& value) {
- return (strncmp(key, "api", 3) == 0);
- }
-
- void _apiWebSocketOnConnected(JsonObject& root) {
- root["apiEnabled"] = apiEnabled();
- root["apiKey"] = apiKey();
- root["apiRestFul"] = apiRestFul();
- root["apiRealTime"] = getSetting("apiRealTime", 1 == API_REAL_TIME_VALUES);
- }
-
- }
-
- // -----------------------------------------------------------------------------
- // Public API
- // -----------------------------------------------------------------------------
-
- bool apiEnabled() {
- return getSetting("apiEnabled", 1 == API_ENABLED);
- }
-
- bool apiRestFul() {
- return getSetting("apiRestFul", 1 == API_RESTFUL);
- }
-
- String apiKey() {
- return getSetting("apiKey", API_KEY);
- }
-
- bool apiAuthenticate(AsyncWebServerRequest *request) {
-
- const auto key = apiKey();
- if (!apiEnabled() || !key.length()) {
- DEBUG_MSG_P(PSTR("[WEBSERVER] HTTP API is not enabled\n"));
- request->send(403);
- return false;
- }
-
- AsyncWebParameter* keyParam = request->getParam("apikey", (request->method() == HTTP_PUT));
- if (!keyParam || !keyParam->value().equals(key)) {
- DEBUG_MSG_P(PSTR("[WEBSERVER] Wrong / missing apikey parameter\n"));
- request->send(403);
- return false;
- }
-
- return true;
-
- }
-
- void apiCommonSetup() {
- wsRegister()
- .onVisible([](JsonObject& root) { root["apiVisible"] = 1; })
- .onConnected(_apiWebSocketOnConnected)
- .onKeyCheck(_apiWebSocketOnKeyCheck);
- }
-
- #endif // WEB_SUPPORT == 1
|