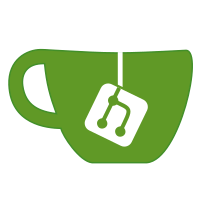 Fix and add unit tests for Caps Word to work with Unicode Map, Auto Shift, Retro Shift. (#17284)
* Fix Caps Word and Unicode Map
* Tests for Caps Word + Auto Shift and Unicode Map.
* Fix formatting
* Add additional keyboard report expectation macros
This commit defines five test utilities, EXPECT_REPORT, EXPECT_UNICODE,
EXPECT_EMPTY_REPORT, EXPECT_ANY_REPORT and EXPECT_NO_REPORT for use with
TestDriver.
EXPECT_REPORT sets a gmock expectation that a given keyboard report will
be sent. For instance,
EXPECT_REPORT(driver, (KC_LSFT, KC_A));
is shorthand for
EXPECT_CALL(driver,
send_keyboard_mock(KeyboardReport(KC_LSFT, KC_A)));
EXPECT_UNICODE sets a gmock expectation that a given Unicode code point
will be sent using UC_LNX input mode. For instance for U+2013,
EXPECT_UNICODE(driver, 0x2013);
expects the sequence of keys:
"Ctrl+Shift+U, 2, 0, 1, 3, space".
EXPECT_EMPTY_REPORT sets a gmock expectation that a given keyboard
report will be sent. For instance
EXPECT_EMPTY_REPORT(driver);
expects a single report without keypresses or modifiers.
EXPECT_ANY_REPORT sets a gmock expectation that a arbitrary keyboard
report will be sent, without matching its contents. For instance
EXPECT_ANY_REPORT(driver).Times(1);
expects a single arbitrary keyboard report will be sent.
EXPECT_NO_REPORT sets a gmock expectation that no keyboard report will
be sent at all.
* Add tap_key() and tap_keys() to TestFixture.
This commit adds a `tap_key(key)` method to TestFixture that taps a
given KeymapKey, optionally with a specified delay between press and
release.
Similarly, the method `tap_keys(key_a, key_b, key_c)` taps a sequence of
KeymapKeys.
* Use EXPECT_REPORT, tap_keys, etc. in most tests.
This commit uses EXPECT_REPORT, EXPECT_UNICODE, EXPECT_EMPTY_REPORT,
EXPECT_NO_REPORT, tap_key() and tap_keys() test utilities from the
previous two commits in most tests. Particularly the EXPECT_REPORT
macro is frequently useful and makes a nice reduction in boilerplate
needed to express many tests.
Co-authored-by: David Kosorin <david@kosorin.net> 1 year ago 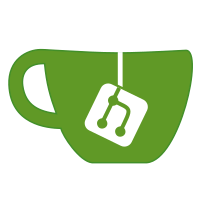 Fix and add unit tests for Caps Word to work with Unicode Map, Auto Shift, Retro Shift. (#17284)
* Fix Caps Word and Unicode Map
* Tests for Caps Word + Auto Shift and Unicode Map.
* Fix formatting
* Add additional keyboard report expectation macros
This commit defines five test utilities, EXPECT_REPORT, EXPECT_UNICODE,
EXPECT_EMPTY_REPORT, EXPECT_ANY_REPORT and EXPECT_NO_REPORT for use with
TestDriver.
EXPECT_REPORT sets a gmock expectation that a given keyboard report will
be sent. For instance,
EXPECT_REPORT(driver, (KC_LSFT, KC_A));
is shorthand for
EXPECT_CALL(driver,
send_keyboard_mock(KeyboardReport(KC_LSFT, KC_A)));
EXPECT_UNICODE sets a gmock expectation that a given Unicode code point
will be sent using UC_LNX input mode. For instance for U+2013,
EXPECT_UNICODE(driver, 0x2013);
expects the sequence of keys:
"Ctrl+Shift+U, 2, 0, 1, 3, space".
EXPECT_EMPTY_REPORT sets a gmock expectation that a given keyboard
report will be sent. For instance
EXPECT_EMPTY_REPORT(driver);
expects a single report without keypresses or modifiers.
EXPECT_ANY_REPORT sets a gmock expectation that a arbitrary keyboard
report will be sent, without matching its contents. For instance
EXPECT_ANY_REPORT(driver).Times(1);
expects a single arbitrary keyboard report will be sent.
EXPECT_NO_REPORT sets a gmock expectation that no keyboard report will
be sent at all.
* Add tap_key() and tap_keys() to TestFixture.
This commit adds a `tap_key(key)` method to TestFixture that taps a
given KeymapKey, optionally with a specified delay between press and
release.
Similarly, the method `tap_keys(key_a, key_b, key_c)` taps a sequence of
KeymapKeys.
* Use EXPECT_REPORT, tap_keys, etc. in most tests.
This commit uses EXPECT_REPORT, EXPECT_UNICODE, EXPECT_EMPTY_REPORT,
EXPECT_NO_REPORT, tap_key() and tap_keys() test utilities from the
previous two commits in most tests. Particularly the EXPECT_REPORT
macro is frequently useful and makes a nice reduction in boilerplate
needed to express many tests.
Co-authored-by: David Kosorin <david@kosorin.net> 1 year ago |
|
-
- /* Copyright 2021 Stefan Kerkmann
- *
- * This program is free software: you can redistribute it and/or modify
- * it under the terms of the GNU General Public License as published by
- * the Free Software Foundation, either version 2 of the License, or
- * (at your option) any later version.
- *
- * This program is distributed in the hope that it will be useful,
- * but WITHOUT ANY WARRANTY; without even the implied warranty of
- * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
- * GNU General Public License for more details.
- *
- * You should have received a copy of the GNU General Public License
- * along with this program. If not, see <http://www.gnu.org/licenses/>.
- */
-
- #include "keyboard_report_util.hpp"
- #include "keycode.h"
- #include "test_common.hpp"
- #include "action_tapping.h"
- #include "test_fixture.hpp"
- #include "test_keymap_key.hpp"
-
- using testing::_;
- using testing::InSequence;
-
- class RetroTapping : public TestFixture {};
-
- TEST_F(RetroTapping, tap_and_hold_mod_tap_hold_key) {
- TestDriver driver;
- InSequence s;
- auto mod_tap_hold_key = KeymapKey(0, 1, 0, SFT_T(KC_P));
-
- set_keymap({mod_tap_hold_key});
-
- /* Press mod-tap-hold key. */
- EXPECT_NO_REPORT(driver);
- mod_tap_hold_key.press();
- idle_for(TAPPING_TERM);
- testing::Mock::VerifyAndClearExpectations(&driver);
-
- /* Release mod-tap-hold key. */
- /* TODO: Why is LSHIFT send at all? */
- EXPECT_REPORT(driver, (KC_LEFT_SHIFT));
- EXPECT_EMPTY_REPORT(driver);
- EXPECT_REPORT(driver, (KC_P));
- EXPECT_EMPTY_REPORT(driver);
- mod_tap_hold_key.release();
- run_one_scan_loop();
- testing::Mock::VerifyAndClearExpectations(&driver);
- }
|