diff --git a/keyboards/takashicompany/center_enter/center_enter.c b/keyboards/takashicompany/center_enter/center_enter.c
new file mode 100644
index 00000000000..4973e6a643e
--- /dev/null
+++ b/keyboards/takashicompany/center_enter/center_enter.c
@@ -0,0 +1,17 @@
+/* Copyright 2021 takashicompany
+ *
+ * This program is free software: you can redistribute it and/or modify
+ * it under the terms of the GNU General Public License as published by
+ * the Free Software Foundation, either version 2 of the License, or
+ * (at your option) any later version.
+ *
+ * This program is distributed in the hope that it will be useful,
+ * but WITHOUT ANY WARRANTY; without even the implied warranty of
+ * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
+ * GNU General Public License for more details.
+ *
+ * You should have received a copy of the GNU General Public License
+ * along with this program. If not, see .
+ */
+
+#include "center_enter.h"
diff --git a/keyboards/takashicompany/center_enter/center_enter.h b/keyboards/takashicompany/center_enter/center_enter.h
new file mode 100644
index 00000000000..0bd576f4328
--- /dev/null
+++ b/keyboards/takashicompany/center_enter/center_enter.h
@@ -0,0 +1,37 @@
+/* Copyright 2021 takashicompany
+ *
+ * This program is free software: you can redistribute it and/or modify
+ * it under the terms of the GNU General Public License as published by
+ * the Free Software Foundation, either version 2 of the License, or
+ * (at your option) any later version.
+ *
+ * This program is distributed in the hope that it will be useful,
+ * but WITHOUT ANY WARRANTY; without even the implied warranty of
+ * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
+ * GNU General Public License for more details.
+ *
+ * You should have received a copy of the GNU General Public License
+ * along with this program. If not, see .
+ */
+
+#pragma once
+
+#include "quantum.h"
+
+/* This is a shortcut to help you visually see your layout.
+ *
+ * The first section contains all of the arguments representing the physical
+ * layout of the board and position of the keys.
+ *
+ * The second converts the arguments into a two-dimensional array which
+ * represents the switch matrix.
+ */
+#define LAYOUT( \
+ k00, k01, k02, k03, k04, k05, k07, k08, k09, k0a, k0b, \
+ k10, k11, k12, k13, k14, k15, k16, k17, k18, k19, k1a, k1b, \
+ k20, k21, k22, k23, k24, k25, k26, k27, k28, k29, k2a, k2b \
+) { \
+ { k00, k01, k02, k03, k04, k05, KC_NO, k07, k08, k09, k0a, k0b }, \
+ { k10, k11, k12, k13, k14, k15, k16, k17, k18, k19, k1a, k1b }, \
+ { k20, k21, k22, k23, k24, k25, k26, k27, k28, k29, k2a, k2b } \
+}
diff --git a/keyboards/takashicompany/center_enter/config.h b/keyboards/takashicompany/center_enter/config.h
new file mode 100644
index 00000000000..94868bde1e7
--- /dev/null
+++ b/keyboards/takashicompany/center_enter/config.h
@@ -0,0 +1,153 @@
+/*
+Copyright 2021 takashicompany
+
+This program is free software: you can redistribute it and/or modify
+it under the terms of the GNU General Public License as published by
+the Free Software Foundation, either version 2 of the License, or
+(at your option) any later version.
+
+This program is distributed in the hope that it will be useful,
+but WITHOUT ANY WARRANTY; without even the implied warranty of
+MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
+GNU General Public License for more details.
+
+You should have received a copy of the GNU General Public License
+along with this program. If not, see .
+*/
+
+#pragma once
+
+#include "config_common.h"
+
+/* USB Device descriptor parameter */
+#define VENDOR_ID 0x7463 // tc = takashicompany
+#define PRODUCT_ID 0x0012
+#define DEVICE_VER 0x0001
+#define MANUFACTURER takashicompany
+#define PRODUCT Center x Enter
+
+/* key matrix size */
+#define MATRIX_ROWS 3
+#define MATRIX_COLS 12
+
+/*
+ * Keyboard Matrix Assignments
+ *
+ * Change this to how you wired your keyboard
+ * COLS: AVR pins used for columns, left to right
+ * ROWS: AVR pins used for rows, top to bottom
+ * DIODE_DIRECTION: COL2ROW = COL = Anode (+), ROW = Cathode (-, marked on diode)
+ * ROW2COL = ROW = Anode (+), COL = Cathode (-, marked on diode)
+ *
+ */
+#define MATRIX_ROW_PINS { E6, B4, B5 }
+#define MATRIX_COL_PINS { F4, F5, F6, F7, B1, B3, D7, B2, B6, D0, D4, C6}
+#define UNUSED_PINS
+
+/* COL2ROW, ROW2COL */
+#define DIODE_DIRECTION COL2ROW
+
+#define ENCODER_RESOLUTION 1
+#define ENCODERS_PAD_A { D2 }
+#define ENCODERS_PAD_B { D1 }
+
+/*
+ * Split Keyboard specific options, make sure you have 'SPLIT_KEYBOARD = yes' in your rules.mk, and define SOFT_SERIAL_PIN.
+ */
+#define SOFT_SERIAL_PIN D0 // or D1, D2, D3, E6
+
+//#define LED_NUM_LOCK_PIN B0
+//#define LED_CAPS_LOCK_PIN B1
+//#define LED_SCROLL_LOCK_PIN B2
+//#define LED_COMPOSE_PIN B3
+//#define LED_KANA_PIN B4
+
+//#define BACKLIGHT_PIN B7
+//#define BACKLIGHT_LEVELS 3
+//#define BACKLIGHT_BREATHING
+
+#define RGB_DI_PIN D3
+#ifdef RGB_DI_PIN
+# define RGBLED_NUM 16
+# define RGBLIGHT_HUE_STEP 8
+# define RGBLIGHT_SAT_STEP 8
+# define RGBLIGHT_VAL_STEP 8
+# define RGBLIGHT_LIMIT_VAL 255 /* The maximum brightness level */
+# define RGBLIGHT_SLEEP /* If defined, the RGB lighting will be switched off when the host goes to sleep */
+# define RGBLIGHT_EFFECT_BREATHING
+# define RGBLIGHT_EFFECT_RAINBOW_MOOD
+# define RGBLIGHT_EFFECT_RAINBOW_SWIRL
+# define RGBLIGHT_EFFECT_SNAKE
+# define RGBLIGHT_EFFECT_KNIGHT
+# define RGBLIGHT_EFFECT_CHRISTMAS
+# define RGBLIGHT_EFFECT_STATIC_GRADIENT
+# define RGBLIGHT_EFFECT_RGB_TEST
+# define RGBLIGHT_EFFECT_ALTERNATING
+/*== customize breathing effect ==*/
+/*==== (DEFAULT) use fixed table instead of exp() and sin() ====*/
+//# define RGBLIGHT_BREATHE_TABLE_SIZE 256 // 256(default) or 128 or 64
+/*==== use exp() and sin() ====*/
+//# define RGBLIGHT_EFFECT_BREATHE_CENTER 1.85 // 1 to 2.7
+//# define RGBLIGHT_EFFECT_BREATHE_MAX 255 // 0 to 255
+#endif
+
+/* Debounce reduces chatter (unintended double-presses) - set 0 if debouncing is not needed */
+#define DEBOUNCE 5
+
+/* define if matrix has ghost (lacks anti-ghosting diodes) */
+//#define MATRIX_HAS_GHOST
+
+/* Mechanical locking support. Use KC_LCAP, KC_LNUM or KC_LSCR instead in keymap */
+#define LOCKING_SUPPORT_ENABLE
+/* Locking resynchronize hack */
+#define LOCKING_RESYNC_ENABLE
+
+/* If defined, GRAVE_ESC will always act as ESC when CTRL is held.
+ * This is useful for the Windows task manager shortcut (ctrl+shift+esc).
+ */
+//#define GRAVE_ESC_CTRL_OVERRIDE
+
+/*
+ * Force NKRO
+ *
+ * Force NKRO (nKey Rollover) to be enabled by default, regardless of the saved
+ * state in the bootmagic EEPROM settings. (Note that NKRO must be enabled in the
+ * makefile for this to work.)
+ *
+ * If forced on, NKRO can be disabled via magic key (default = LShift+RShift+N)
+ * until the next keyboard reset.
+ *
+ * NKRO may prevent your keystrokes from being detected in the BIOS, but it is
+ * fully operational during normal computer usage.
+ *
+ * For a less heavy-handed approach, enable NKRO via magic key (LShift+RShift+N)
+ * or via bootmagic (hold SPACE+N while plugging in the keyboard). Once set by
+ * bootmagic, NKRO mode will always be enabled until it is toggled again during a
+ * power-up.
+ *
+ */
+//#define FORCE_NKRO
+
+/*
+ * Feature disable options
+ * These options are also useful to firmware size reduction.
+ */
+
+/* disable debug print */
+//#define NO_DEBUG
+
+/* disable print */
+//#define NO_PRINT
+
+/* disable action features */
+//#define NO_ACTION_LAYER
+//#define NO_ACTION_TAPPING
+//#define NO_ACTION_ONESHOT
+
+/* disable these deprecated features by default */
+#define NO_ACTION_MACRO
+#define NO_ACTION_FUNCTION
+
+/* Bootmagic Lite key configuration */
+//#define BOOTMAGIC_LITE_ROW 0
+//#define BOOTMAGIC_LITE_COLUMN 0
diff --git a/keyboards/takashicompany/center_enter/info.json b/keyboards/takashicompany/center_enter/info.json
new file mode 100644
index 00000000000..f0f3af1f390
--- /dev/null
+++ b/keyboards/takashicompany/center_enter/info.json
@@ -0,0 +1,191 @@
+{
+ "keyboard_name": "Center x Enter",
+ "url": "",
+ "maintainer": "takashicompany",
+ "layouts": {
+ "LAYOUT": {
+ "layout": [
+ {
+ "label": "201",
+ "x": 0,
+ "y": 0,
+ "w": 1.5
+ },
+ {
+ "label": "1",
+ "x": 1.5,
+ "y": 0
+ },
+ {
+ "label": "2",
+ "x": 2.5,
+ "y": 0
+ },
+ {
+ "label": "3",
+ "x": 3.5,
+ "y": 0
+ },
+ {
+ "label": "4",
+ "x": 4.5,
+ "y": 0
+ },
+ {
+ "label": "5",
+ "x": 5.5,
+ "y": 0
+ },
+ {
+ "label": "901",
+ "x": 6.75,
+ "y": 0,
+ "w": 1.25,
+ "h": 2
+ },
+ {
+ "label": "6",
+ "x": 8,
+ "y": 0
+ },
+ {
+ "label": "7",
+ "x": 9,
+ "y": 0
+ },
+ {
+ "label": "8",
+ "x": 10,
+ "y": 0
+ },
+ {
+ "label": "9",
+ "x": 11,
+ "y": 0
+ },
+ {
+ "label": "10",
+ "x": 12,
+ "y": 0
+ },
+ {
+ "label": "301",
+ "x": 0,
+ "y": 1,
+ "w": 1.75
+ },
+ {
+ "label": "11",
+ "x": 1.75,
+ "y": 1
+ },
+ {
+ "label": "12",
+ "x": 2.75,
+ "y": 1
+ },
+ {
+ "label": "13",
+ "x": 3.75,
+ "y": 1
+ },
+ {
+ "label": "14",
+ "x": 4.75,
+ "y": 1
+ },
+ {
+ "label": "15",
+ "x": 5.75,
+ "y": 1
+ },
+ {
+ "label": "16",
+ "x": 8,
+ "y": 1
+ },
+ {
+ "label": "17",
+ "x": 9,
+ "y": 1
+ },
+ {
+ "label": "18",
+ "x": 10,
+ "y": 1
+ },
+ {
+ "label": "19",
+ "x": 11,
+ "y": 1
+ },
+ {
+ "label": "20",
+ "x": 12,
+ "y": 1
+ },
+ {
+ "label": "401",
+ "x": 0,
+ "y": 2,
+ "w": 2
+ },
+ {
+ "label": "21",
+ "x": 2,
+ "y": 2
+ },
+ {
+ "label": "22",
+ "x": 3,
+ "y": 2
+ },
+ {
+ "label": "23",
+ "x": 4,
+ "y": 2
+ },
+ {
+ "label": "24",
+ "x": 5,
+ "y": 2
+ },
+ {
+ "label": "25",
+ "x": 6,
+ "y": 2
+ },
+ {
+ "label": "31",
+ "x": 7,
+ "y": 2
+ },
+ {
+ "label": "26",
+ "x": 8,
+ "y": 2
+ },
+ {
+ "label": "27",
+ "x": 9,
+ "y": 2
+ },
+ {
+ "label": "28",
+ "x": 10,
+ "y": 2
+ },
+ {
+ "label": "29",
+ "x": 11,
+ "y": 2
+ },
+ {
+ "label": "30",
+ "x": 12,
+ "y": 2
+ }
+ ]
+ }
+ }
+}
\ No newline at end of file
diff --git a/keyboards/takashicompany/center_enter/keymaps/default/keymap.c b/keyboards/takashicompany/center_enter/keymaps/default/keymap.c
new file mode 100644
index 00000000000..1003d0558cf
--- /dev/null
+++ b/keyboards/takashicompany/center_enter/keymaps/default/keymap.c
@@ -0,0 +1,97 @@
+/* Copyright 2021 takashicompany
+ *
+ * This program is free software: you can redistribute it and/or modify
+ * it under the terms of the GNU General Public License as published by
+ * the Free Software Foundation, either version 2 of the License, or
+ * (at your option) any later version.
+ *
+ * This program is distributed in the hope that it will be useful,
+ * but WITHOUT ANY WARRANTY; without even the implied warranty of
+ * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
+ * GNU General Public License for more details.
+ *
+ * You should have received a copy of the GNU General Public License
+ * along with this program. If not, see .
+ */
+#include QMK_KEYBOARD_H
+
+// Defines the keycodes used by our macros in process_record_user
+enum custom_keycodes {
+ SWITCH_LANG
+};
+
+const uint16_t PROGMEM keymaps[][MATRIX_ROWS][MATRIX_COLS] = {
+
+ LAYOUT(
+ KC_TAB, LT(2, KC_Q), KC_W, KC_E, KC_R, KC_T, KC_Y, KC_U, KC_I, KC_O, ALT_T(KC_P),
+ KC_LCTL, KC_A, KC_S, LT(2, KC_D), KC_F, KC_G, SWITCH_LANG, KC_H, KC_J, LT(2, KC_K), KC_L, KC_ENT,
+ KC_LSFT, KC_Z, GUI_T(KC_X), ALT_T(KC_C), LT(1, KC_V), KC_B, KC_SPC, KC_N, LT(1, KC_M), KC_COMM, CTL_T(KC_DOT), KC_BSPC
+ ),
+
+ LAYOUT(
+ KC_TRNS, KC_1, KC_2, KC_3, KC_4, KC_5, KC_6, KC_7, KC_8, KC_9, KC_0,
+ KC_TRNS, KC_EQL, KC_LBRC, KC_SLSH, KC_MINS, KC_RO, KC_TRNS, KC_SCLN, KC_QUOT, KC_RBRC, KC_NUHS, KC_JYEN,
+ KC_TRNS, KC_LSFT, KC_LGUI, KC_LALT, KC_LANG2, KC_LSFT, KC_TRNS, KC_SPC, KC_LANG1, KC_TRNS, KC_TRNS, KC_DEL
+ ),
+
+ LAYOUT(
+ KC_TRNS, KC_ESC, KC_TAB, KC_NO, KC_NO, KC_NO, KC_NO, KC_NO, KC_UP, KC_NO, KC_NO,
+ KC_TRNS, KC_LCTL, KC_TRNS, KC_QUES, KC_EXLM, KC_NO, KC_TRNS, KC_NO, KC_LEFT, KC_DOWN, KC_RGHT, KC_NO,
+ KC_TRNS, KC_LSFT, KC_LGUI, KC_LALT, KC_LANG2, KC_TRNS, KC_TRNS, KC_TRNS, KC_LANG1, KC_NO, MO(3), KC_DEL
+ ),
+
+ LAYOUT(
+ KC_TRNS, RGB_TOG, RGB_MOD, RGB_HUI, RGB_SAI, RGB_VAI, KC_F1, KC_F2, KC_F3, KC_F4, KC_F5,
+ KC_TRNS, RGB_M_P, RGB_M_B, RGB_M_R, RGB_M_SW, RGB_M_SN, KC_TRNS, KC_F6, KC_F7, KC_F8, KC_F9, KC_F10,
+ KC_TRNS, RGB_M_K, RGB_M_X, RGB_M_G, RGB_M_T, RGB_M_T, KC_TRNS, KC_F11, KC_F12, KC_CAPS, KC_NO, KC_NO
+ ),
+
+ LAYOUT(
+ KC_NO, KC_NO, KC_NO, KC_NO, KC_NO, KC_NO, KC_NO, KC_NO, KC_NO, KC_NO, KC_NO,
+ KC_NO, KC_NO, KC_NO, KC_NO, KC_NO, KC_NO, KC_NO, KC_NO, KC_NO, KC_NO, KC_NO, KC_NO,
+ KC_NO, KC_NO, KC_NO, KC_NO, KC_NO, KC_NO, KC_NO, KC_NO, KC_NO, KC_NO, KC_NO, KC_NO
+ ),
+};
+
+bool is_lang1 = false;
+
+bool process_record_user(uint16_t keycode, keyrecord_t *record) {
+ switch (keycode) {
+ case SWITCH_LANG:
+ if (record->event.pressed) {
+
+ if (is_lang1) {
+ register_code(KC_LANG1);
+ is_lang1 = false;
+ }
+ else {
+ register_code(KC_LANG2);
+ is_lang1 = true;
+ }
+ }
+ break;
+ case KC_LANG1:
+ if (record->event.pressed) {
+ is_lang1 = false;
+ }
+ break;
+
+ case KC_LANG2:
+ if (record->event.pressed) {
+ is_lang1 = true;
+ }
+ break;
+ }
+ return true;
+}
+
+bool encoder_update_user(uint8_t index, bool clockwise) {
+
+ if (clockwise) {
+ tap_code(KC_MS_WH_UP);
+ } else {
+ tap_code(KC_MS_WH_DOWN);
+ }
+
+ return true;
+}
diff --git a/keyboards/takashicompany/center_enter/keymaps/via/config.h b/keyboards/takashicompany/center_enter/keymaps/via/config.h
new file mode 100644
index 00000000000..ec7c614382d
--- /dev/null
+++ b/keyboards/takashicompany/center_enter/keymaps/via/config.h
@@ -0,0 +1 @@
+#define DYNAMIC_KEYMAP_LAYER_COUNT 8
\ No newline at end of file
diff --git a/keyboards/takashicompany/center_enter/keymaps/via/keymap.c b/keyboards/takashicompany/center_enter/keymaps/via/keymap.c
new file mode 100644
index 00000000000..cc11ca18a81
--- /dev/null
+++ b/keyboards/takashicompany/center_enter/keymaps/via/keymap.c
@@ -0,0 +1,91 @@
+/* Copyright 2021 takashicompany
+ *
+ * This program is free software: you can redistribute it and/or modify
+ * it under the terms of the GNU General Public License as published by
+ * the Free Software Foundation, either version 2 of the License, or
+ * (at your option) any later version.
+ *
+ * This program is distributed in the hope that it will be useful,
+ * but WITHOUT ANY WARRANTY; without even the implied warranty of
+ * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
+ * GNU General Public License for more details.
+ *
+ * You should have received a copy of the GNU General Public License
+ * along with this program. If not, see .
+ */
+#include QMK_KEYBOARD_H
+
+// Defines the keycodes used by our macros in process_record_user
+enum custom_keycodes {
+ SWITCH_LANG
+};
+
+const uint16_t PROGMEM keymaps[][MATRIX_ROWS][MATRIX_COLS] = {
+
+ LAYOUT(
+ KC_TAB, LT(2, KC_Q), KC_W, KC_E, KC_R, KC_T, KC_Y, KC_U, KC_I, KC_O, ALT_T(KC_P),
+ KC_LCTL, KC_A, KC_S, LT(2, KC_D), KC_F, KC_G, SWITCH_LANG, KC_H, KC_J, LT(2, KC_K), KC_L, KC_ENT,
+ KC_LSFT, KC_Z, GUI_T(KC_X), ALT_T(KC_C), LT(1, KC_V), KC_B, KC_SPC, KC_N, LT(1, KC_M), KC_COMM, CTL_T(KC_DOT), KC_BSPC
+ ),
+
+ LAYOUT(
+ KC_TRNS, KC_1, KC_2, KC_3, KC_4, KC_5, KC_6, KC_7, KC_8, KC_9, KC_0,
+ KC_TRNS, KC_EQL, KC_LBRC, KC_SLSH, KC_MINS, KC_RO, KC_TRNS, KC_SCLN, KC_QUOT, KC_RBRC, KC_NUHS, KC_JYEN,
+ KC_TRNS, KC_LSFT, KC_LGUI, KC_LALT, KC_LANG2, KC_LSFT, KC_TRNS, KC_SPC, KC_LANG1, KC_TRNS, KC_TRNS, KC_DEL
+ ),
+
+ LAYOUT(
+ KC_TRNS, KC_ESC, KC_TAB, KC_NO, KC_NO, KC_NO, KC_NO, KC_NO, KC_UP, KC_NO, KC_NO,
+ KC_TRNS, KC_LCTL, KC_TRNS, KC_QUES, KC_EXLM, KC_NO, KC_TRNS, KC_NO, KC_LEFT, KC_DOWN, KC_RGHT, KC_NO,
+ KC_TRNS, KC_LSFT, KC_LGUI, KC_LALT, KC_LANG2, KC_TRNS, KC_TRNS, KC_TRNS, KC_LANG1, KC_NO, MO(3), KC_DEL
+ ),
+
+ LAYOUT(
+ KC_TRNS, RGB_TOG, RGB_MOD, RGB_HUI, RGB_SAI, RGB_VAI, KC_F1, KC_F2, KC_F3, KC_F4, KC_F5,
+ KC_TRNS, RGB_M_P, RGB_M_B, RGB_M_R, RGB_M_SW, RGB_M_SN, KC_TRNS, KC_F6, KC_F7, KC_F8, KC_F9, KC_F10,
+ KC_TRNS, RGB_M_K, RGB_M_X, RGB_M_G, RGB_M_T, RGB_M_T, KC_TRNS, KC_F11, KC_F12, KC_CAPS, KC_NO, KC_NO
+ )
+};
+
+bool is_lang1 = false;
+
+bool process_record_user(uint16_t keycode, keyrecord_t *record) {
+ switch (keycode) {
+ case SWITCH_LANG:
+ if (record->event.pressed) {
+
+ if (is_lang1) {
+ register_code(KC_LANG1);
+ is_lang1 = false;
+ }
+ else {
+ register_code(KC_LANG2);
+ is_lang1 = true;
+ }
+ }
+ break;
+ case KC_LANG1:
+ if (record->event.pressed) {
+ is_lang1 = false;
+ }
+ break;
+
+ case KC_LANG2:
+ if (record->event.pressed) {
+ is_lang1 = true;
+ }
+ break;
+ }
+ return true;
+}
+
+bool encoder_update_user(uint8_t index, bool clockwise) {
+
+ if (clockwise) {
+ tap_code(KC_MS_WH_UP);
+ } else {
+ tap_code(KC_MS_WH_DOWN);
+ }
+
+ return true;
+}
diff --git a/keyboards/takashicompany/center_enter/keymaps/via/rules.mk b/keyboards/takashicompany/center_enter/keymaps/via/rules.mk
new file mode 100644
index 00000000000..036bd6d1c3e
--- /dev/null
+++ b/keyboards/takashicompany/center_enter/keymaps/via/rules.mk
@@ -0,0 +1 @@
+VIA_ENABLE = yes
\ No newline at end of file
diff --git a/keyboards/takashicompany/center_enter/readme.md b/keyboards/takashicompany/center_enter/readme.md
new file mode 100644
index 00000000000..d8db5cc7eb3
--- /dev/null
+++ b/keyboards/takashicompany/center_enter/readme.md
@@ -0,0 +1,29 @@
+# Center x Enter
+
+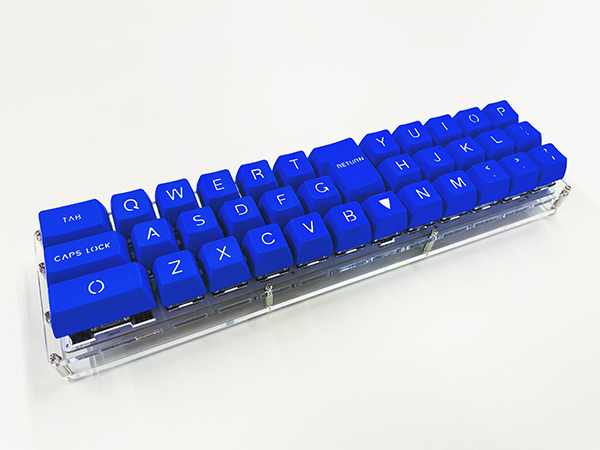
+
+A 30% keyboard with the enter key placed in the center.
+Option to install a rotary encoder is also available.
+VIA (Remap) is also supported.
+
+* Keyboard Maintainer: [takashicompany](https://github.com/takashicompany)
+* Hardware Supported: Center x Enter PCB, Pro Micro
+* Hardware Availability: https://github.com/takashicompany/center-enter
+
+Make example for this keyboard (after setting up your build environment):
+
+ make takashicompany/center_enter:default
+
+Flashing example for this keyboard:
+
+ make takashicompany/center_enter:default:flash
+
+See the [build environment setup](https://docs.qmk.fm/#/getting_started_build_tools) and the [make instructions](https://docs.qmk.fm/#/getting_started_make_guide) for more information. Brand new to QMK? Start with our [Complete Newbs Guide](https://docs.qmk.fm/#/newbs).
+
+## Bootloader
+
+Enter the bootloader in 3 ways:
+
+* **Bootmagic reset**: Hold down the key at (0,0) in the matrix (usually the top left key or Escape) and plug in the keyboard
+* **Physical reset button**: Briefly press the button on the back of the PCB - some may have pads you must short instead
+* **Keycode in layout**: Press the key mapped to `RESET` if it is available
diff --git a/keyboards/takashicompany/center_enter/rules.mk b/keyboards/takashicompany/center_enter/rules.mk
new file mode 100644
index 00000000000..d8e321ce2ec
--- /dev/null
+++ b/keyboards/takashicompany/center_enter/rules.mk
@@ -0,0 +1,23 @@
+# MCU name
+MCU = atmega32u4
+
+# Bootloader selection
+BOOTLOADER = caterina
+
+# Build Options
+# change yes to no to disable
+#
+BOOTMAGIC_ENABLE = yes # Enable Bootmagic Lite
+MOUSEKEY_ENABLE = yes # Mouse keys
+EXTRAKEY_ENABLE = yes # Audio control and System control
+CONSOLE_ENABLE = no # Console for debug
+COMMAND_ENABLE = no # Commands for debug and configuration
+# Do not enable SLEEP_LED_ENABLE. it uses the same timer as BACKLIGHT_ENABLE
+SLEEP_LED_ENABLE = no # Breathing sleep LED during USB suspend
+# if this doesn't work, see here: https://github.com/tmk/tmk_keyboard/wiki/FAQ#nkro-doesnt-work
+NKRO_ENABLE = no # USB Nkey Rollover
+BACKLIGHT_ENABLE = no # Enable keyboard backlight functionality
+RGBLIGHT_ENABLE = yes # Enable keyboard RGB underglow
+AUDIO_ENABLE = no # Audio output
+
+ENCODER_ENABLE = yes