@ -0,0 +1,21 @@ | |||
/* | |||
Copyright 2012 Jun Wako <wakojun@gmail.com> | |||
Copyright 2015 Jack Humbert | |||
This program is free software: you can redistribute it and/or modify | |||
it under the terms of the GNU General Public License as published by | |||
the Free Software Foundation, either version 2 of the License, or | |||
(at your option) any later version. | |||
This program is distributed in the hope that it will be useful, | |||
but WITHOUT ANY WARRANTY; without even the implied warranty of | |||
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the | |||
GNU General Public License for more details. | |||
You should have received a copy of the GNU General Public License | |||
along with this program. If not, see <http://www.gnu.org/licenses/>. | |||
*/ | |||
#pragma once | |||
#include "serial_config.h" |
@ -0,0 +1,162 @@ | |||
#include <util/twi.h> | |||
#include <avr/io.h> | |||
#include <stdlib.h> | |||
#include <avr/interrupt.h> | |||
#include <util/twi.h> | |||
#include <stdbool.h> | |||
#include "i2c.h" | |||
#ifdef USE_I2C | |||
// Limits the amount of we wait for any one i2c transaction. | |||
// Since were running SCL line 100kHz (=> 10μs/bit), and each transactions is | |||
// 9 bits, a single transaction will take around 90μs to complete. | |||
// | |||
// (F_CPU/SCL_CLOCK) => # of μC cycles to transfer a bit | |||
// poll loop takes at least 8 clock cycles to execute | |||
#define I2C_LOOP_TIMEOUT (9+1)*(F_CPU/SCL_CLOCK)/8 | |||
#define BUFFER_POS_INC() (slave_buffer_pos = (slave_buffer_pos+1)%SLAVE_BUFFER_SIZE) | |||
volatile uint8_t i2c_slave_buffer[SLAVE_BUFFER_SIZE]; | |||
static volatile uint8_t slave_buffer_pos; | |||
static volatile bool slave_has_register_set = false; | |||
// Wait for an i2c operation to finish | |||
inline static | |||
void i2c_delay(void) { | |||
uint16_t lim = 0; | |||
while(!(TWCR & (1<<TWINT)) && lim < I2C_LOOP_TIMEOUT) | |||
lim++; | |||
// easier way, but will wait slightly longer | |||
// _delay_us(100); | |||
} | |||
// Setup twi to run at 100kHz | |||
void i2c_master_init(void) { | |||
// no prescaler | |||
TWSR = 0; | |||
// Set TWI clock frequency to SCL_CLOCK. Need TWBR>10. | |||
// Check datasheets for more info. | |||
TWBR = ((F_CPU/SCL_CLOCK)-16)/2; | |||
} | |||
// Start a transaction with the given i2c slave address. The direction of the | |||
// transfer is set with I2C_READ and I2C_WRITE. | |||
// returns: 0 => success | |||
// 1 => error | |||
uint8_t i2c_master_start(uint8_t address) { | |||
TWCR = (1<<TWINT) | (1<<TWEN) | (1<<TWSTA); | |||
i2c_delay(); | |||
// check that we started successfully | |||
if ( (TW_STATUS != TW_START) && (TW_STATUS != TW_REP_START)) | |||
return 1; | |||
TWDR = address; | |||
TWCR = (1<<TWINT) | (1<<TWEN); | |||
i2c_delay(); | |||
if ( (TW_STATUS != TW_MT_SLA_ACK) && (TW_STATUS != TW_MR_SLA_ACK) ) | |||
return 1; // slave did not acknowledge | |||
else | |||
return 0; // success | |||
} | |||
// Finish the i2c transaction. | |||
void i2c_master_stop(void) { | |||
TWCR = (1<<TWINT) | (1<<TWEN) | (1<<TWSTO); | |||
uint16_t lim = 0; | |||
while(!(TWCR & (1<<TWSTO)) && lim < I2C_LOOP_TIMEOUT) | |||
lim++; | |||
} | |||
// Write one byte to the i2c slave. | |||
// returns 0 => slave ACK | |||
// 1 => slave NACK | |||
uint8_t i2c_master_write(uint8_t data) { | |||
TWDR = data; | |||
TWCR = (1<<TWINT) | (1<<TWEN); | |||
i2c_delay(); | |||
// check if the slave acknowledged us | |||
return (TW_STATUS == TW_MT_DATA_ACK) ? 0 : 1; | |||
} | |||
// Read one byte from the i2c slave. If ack=1 the slave is acknowledged, | |||
// if ack=0 the acknowledge bit is not set. | |||
// returns: byte read from i2c device | |||
uint8_t i2c_master_read(int ack) { | |||
TWCR = (1<<TWINT) | (1<<TWEN) | (ack<<TWEA); | |||
i2c_delay(); | |||
return TWDR; | |||
} | |||
void i2c_reset_state(void) { | |||
TWCR = 0; | |||
} | |||
void i2c_slave_init(uint8_t address) { | |||
TWAR = address << 0; // slave i2c address | |||
// TWEN - twi enable | |||
// TWEA - enable address acknowledgement | |||
// TWINT - twi interrupt flag | |||
// TWIE - enable the twi interrupt | |||
TWCR = (1<<TWIE) | (1<<TWEA) | (1<<TWINT) | (1<<TWEN); | |||
} | |||
ISR(TWI_vect); | |||
ISR(TWI_vect) { | |||
uint8_t ack = 1; | |||
switch(TW_STATUS) { | |||
case TW_SR_SLA_ACK: | |||
// this device has been addressed as a slave receiver | |||
slave_has_register_set = false; | |||
break; | |||
case TW_SR_DATA_ACK: | |||
// this device has received data as a slave receiver | |||
// The first byte that we receive in this transaction sets the location | |||
// of the read/write location of the slaves memory that it exposes over | |||
// i2c. After that, bytes will be written at slave_buffer_pos, incrementing | |||
// slave_buffer_pos after each write. | |||
if(!slave_has_register_set) { | |||
slave_buffer_pos = TWDR; | |||
// don't acknowledge the master if this memory loctaion is out of bounds | |||
if ( slave_buffer_pos >= SLAVE_BUFFER_SIZE ) { | |||
ack = 0; | |||
slave_buffer_pos = 0; | |||
} | |||
slave_has_register_set = true; | |||
} else { | |||
i2c_slave_buffer[slave_buffer_pos] = TWDR; | |||
BUFFER_POS_INC(); | |||
} | |||
break; | |||
case TW_ST_SLA_ACK: | |||
case TW_ST_DATA_ACK: | |||
// master has addressed this device as a slave transmitter and is | |||
// requesting data. | |||
TWDR = i2c_slave_buffer[slave_buffer_pos]; | |||
BUFFER_POS_INC(); | |||
break; | |||
case TW_BUS_ERROR: // something went wrong, reset twi state | |||
TWCR = 0; | |||
default: | |||
break; | |||
} | |||
// Reset everything, so we are ready for the next TWI interrupt | |||
TWCR |= (1<<TWIE) | (1<<TWINT) | (ack<<TWEA) | (1<<TWEN); | |||
} | |||
#endif |
@ -0,0 +1,49 @@ | |||
#ifndef I2C_H | |||
#define I2C_H | |||
#include <stdint.h> | |||
#ifndef F_CPU | |||
#define F_CPU 16000000UL | |||
#endif | |||
#define I2C_READ 1 | |||
#define I2C_WRITE 0 | |||
#define I2C_ACK 1 | |||
#define I2C_NACK 0 | |||
#define SLAVE_BUFFER_SIZE 0x10 | |||
// i2c SCL clock frequency | |||
#define SCL_CLOCK 400000L | |||
extern volatile uint8_t i2c_slave_buffer[SLAVE_BUFFER_SIZE]; | |||
void i2c_master_init(void); | |||
uint8_t i2c_master_start(uint8_t address); | |||
void i2c_master_stop(void); | |||
uint8_t i2c_master_write(uint8_t data); | |||
uint8_t i2c_master_read(int); | |||
void i2c_reset_state(void); | |||
void i2c_slave_init(uint8_t address); | |||
static inline unsigned char i2c_start_read(unsigned char addr) { | |||
return i2c_master_start((addr << 1) | I2C_READ); | |||
} | |||
static inline unsigned char i2c_start_write(unsigned char addr) { | |||
return i2c_master_start((addr << 1) | I2C_WRITE); | |||
} | |||
// from SSD1306 scrips | |||
extern unsigned char i2c_rep_start(unsigned char addr); | |||
extern void i2c_start_wait(unsigned char addr); | |||
extern unsigned char i2c_readAck(void); | |||
extern unsigned char i2c_readNak(void); | |||
extern unsigned char i2c_read(unsigned char ack); | |||
#define i2c_read(ack) (ack) ? i2c_readAck() : i2c_readNak(); | |||
#endif |
@ -0,0 +1,41 @@ | |||
/* | |||
This is the c configuration file for the keymap | |||
Copyright 2012 Jun Wako <wakojun@gmail.com> | |||
Copyright 2015 Jack Humbert | |||
This program is free software: you can redistribute it and/or modify | |||
it under the terms of the GNU General Public License as published by | |||
the Free Software Foundation, either version 2 of the License, or | |||
(at your option) any later version. | |||
This program is distributed in the hope that it will be useful, | |||
but WITHOUT ANY WARRANTY; without even the implied warranty of | |||
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the | |||
GNU General Public License for more details. | |||
You should have received a copy of the GNU General Public License | |||
along with this program. If not, see <http://www.gnu.org/licenses/>. | |||
*/ | |||
#pragma once | |||
#include "config.h" | |||
/* Use I2C or Serial, not both */ | |||
#define USE_SERIAL | |||
// #define USE_I2C | |||
/* Select hand configuration */ | |||
#define MASTER_LEFT | |||
// #define MASTER_RIGHT | |||
// #define EE_HANDS | |||
// Underglow | |||
/* | |||
#undef RGBLED_NUM | |||
#define RGBLED_NUM 14 // Number of LEDs | |||
#define RGBLIGHT_ANIMATIONS | |||
#define RGBLIGHT_SLEEP | |||
*/ |
@ -0,0 +1,155 @@ | |||
#include QMK_KEYBOARD_H | |||
extern keymap_config_t keymap_config; | |||
#define _QWERTY 0 | |||
#define _LOWER 1 | |||
#define _RAISE 2 | |||
#define _ADJUST 16 | |||
enum custom_keycodes { | |||
QWERTY = SAFE_RANGE, | |||
LOWER, | |||
RAISE, | |||
ADJUST, | |||
}; | |||
const uint16_t PROGMEM keymaps[][MATRIX_ROWS][MATRIX_COLS] = { | |||
/* QWERTY | |||
* ,-----------------------------------------. ,-----------------------------------------. | |||
* | ESC | 1 | 2 | 3 | 4 | 5 | | 6 | 7 | 8 | 9 | 0 | ~ | | |||
* |------+------+------+------+------+------| |------+------+------+------+------+------| | |||
* | Tab | Q | W | E | R | T | | Y | U | I | O | P | - | | |||
* |------+------+------+------+------+------| |------+------+------+------+------+------| | |||
* |LCTRL | A | S | D | F | G |-------. ,-------| H | J | K | L | ; | ' | | |||
* |------+------+------+------+------+------| [ | | ] |------+------+------+------+------+------| | |||
* |LShift| Z | X | C | V | B |-------| |-------| N | M | , | . | / |RShift| | |||
* `-----------------------------------------/ / \ \-----------------------------------------' | |||
* |LOWER | LGUI | Alt | /Space / \Enter \ |BackSP| RGUI |RAISE | | |||
* | | | |/ / \ \ | | | | | |||
* `-------------------''-------' '------''--------------------' | |||
*/ | |||
[_QWERTY] = LAYOUT( \ | |||
KC_ESC, KC_1, KC_2, KC_3, KC_4, KC_5, KC_6, KC_7, KC_8, KC_9, KC_0, KC_GRV, \ | |||
KC_TAB, KC_Q, KC_W, KC_E, KC_R, KC_T, KC_Y, KC_U, KC_I, KC_O, KC_P, KC_MINS, \ | |||
KC_LCTRL, KC_A, KC_S, KC_D, KC_F, KC_G, KC_H, KC_J, KC_K, KC_L, KC_SCLN, KC_QUOT, \ | |||
KC_LSFT, KC_Z, KC_X, KC_C, KC_V, KC_B, KC_LBRC, KC_RBRC, KC_N, KC_M, KC_COMM, KC_DOT, KC_SLSH, KC_RSFT, \ | |||
LOWER,KC_LGUI, KC_LALT, KC_SPC, KC_ENT, KC_BSPC, KC_RGUI, RAISE \ | |||
), | |||
/* LOWER | |||
* ,-----------------------------------------. ,-----------------------------------------. | |||
* | F1 | F2 | F3 | F4 | F5 | F6 | | F7 | F8 | F9 | F10 | F11 | F12 | | |||
* |------+------+------+------+------+------| |------+------+------+------+------+------| | |||
* | ~ | ! | @ | # | $ | % | | ^ | & | * | ( | ) | | | |||
* |------+------+------+------+------+------| |------+------+------+------+------+------| | |||
* | | | | | | |-------. ,-------| | _ | + | | | | | |||
* |------+------+------+------+------+------| [ | | ] |------+------+------+------+------+------| | |||
* | | | | | | |-------| |-------| |ISO ~ |ISO | | | | | | |||
* `-----------------------------------------/ / \ \-----------------------------------------' | |||
* |LOWER | LGUI | Alt | /Space / \Enter \ |BackSP| RGUI |RAISE | | |||
* | | | |/ / \ \ | | | | | |||
* `-------------------''-------' '------''--------------------' | |||
*/ | |||
[_LOWER] = LAYOUT( \ | |||
KC_F1, KC_F2, KC_F3, KC_F4, KC_F5, KC_F6, KC_F7, KC_F8, KC_F9, KC_F10, KC_F11, KC_F12, \ | |||
KC_TILD, KC_EXLM, KC_AT, KC_HASH, KC_DLR, KC_PERC, KC_CIRC, KC_AMPR, KC_ASTR, KC_LPRN, KC_RPRN, _______, \ | |||
_______, _______, _______, _______, _______, _______, _______, KC_UNDS, KC_PLUS, KC_LCBR, KC_RCBR, KC_PIPE, \ | |||
_______, _______, _______, _______, _______, _______, _______, _______, _______,S(KC_NUHS),S(KC_NUBS),_______, _______, _______,\ | |||
_______, _______, _______, _______, _______, _______, _______, _______\ | |||
), | |||
/* RAISE | |||
* ,-----------------------------------------. ,-----------------------------------------. | |||
* | F1 | F2 | F3 | F4 | F5 | F6 | | F7 | F8 | F9 | F10 | F11 | F12 | | |||
* |------+------+------+------+------+------| |------+------+------+------+------+------| | |||
* | ` | 1 | 2 | 3 | 4 | 5 | | 6 | 7 | 8 | 9 | 0 | | | |||
* |------+------+------+------+------+------| |------+------+------+------+------+------| | |||
* | | | | | | |-------. ,-------| | Left | Down | Up |Right | | | |||
* |------+------+------+------+------+------| [ | | ] |------+------+------+------+------+------| | |||
* | | | | | | |-------| |-------| + | - | = | [ | ] | \ | | |||
* `-----------------------------------------/ / \ \-----------------------------------------' | |||
* |LOWER | LGUI | Alt | /Space / \Enter \ |BackSP| RGUI |RAISE | | |||
* | | | |/ / \ \ | | | | | |||
* `-------------------''-------' '------''--------------------' | |||
*/ | |||
[_RAISE] = LAYOUT( \ | |||
KC_F1, KC_F2, KC_F3, KC_F4, KC_F5, KC_F6, KC_F7, KC_F8, KC_F9, KC_F10, KC_F11, KC_F12, \ | |||
KC_GRV, KC_1, KC_2, KC_3, KC_4, KC_5, KC_6, KC_7, KC_8, KC_9, KC_0, _______, \ | |||
_______, _______, _______, _______, _______, _______, XXXXXXX, KC_LEFT, KC_DOWN, KC_UP, KC_RGHT, XXXXXXX, \ | |||
_______, _______, _______, _______, _______, _______, _______, _______, KC_PLUS, KC_MINS, KC_EQL, KC_LBRC, KC_RBRC, KC_BSLS, \ | |||
_______, _______, _______, _______, _______, _______, _______, _______ \ | |||
), | |||
/* ADJUST (Layers for Underglow) | |||
* ,-----------------------------------------. ,-----------------------------------------. | |||
* | | | | | | | | | | | | | | | |||
* |------+------+------+------+------+------| |------+------+------+------+------+------| | |||
* | | | | | | | | | | | | | | | |||
* |------+------+------+------+------+------| |------+------+------+------+------+------| | |||
* | | | | | | |-------. ,-------| | | | | | | | |||
* |------+------+------+------+------+------| | | |------+------+------+------+------+------| | |||
* | | | | | | |-------| |-------| | | | | | | | |||
* `-----------------------------------------/ / \ \-----------------------------------------' | |||
* |LOWER | LGUI | Alt | /Space / \Enter \ |BackSP| RGUI |RAISE | | |||
* | | | |/ / \ \ | | | | | |||
* `-------------------''-------' '------''--------------------' | |||
*/ | |||
[_ADJUST] = LAYOUT( \ | |||
XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, \ | |||
XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, \ | |||
XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, \ | |||
XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX,\ | |||
_______, _______, _______, _______, _______, _______, _______, _______ \ | |||
) | |||
}; | |||
void persistent_default_layer_set(uint16_t default_layer) { | |||
eeconfig_update_default_layer(default_layer); | |||
default_layer_set(default_layer); | |||
} | |||
bool process_record_user(uint16_t keycode, keyrecord_t *record) { | |||
switch (keycode) { | |||
case QWERTY: | |||
if (record->event.pressed) { | |||
print("mode just switched to qwerty and this is a huge string\n"); | |||
set_single_persistent_default_layer(_QWERTY); | |||
} | |||
return false; | |||
break; | |||
case LOWER: | |||
if (record->event.pressed) { | |||
layer_on(_LOWER); | |||
update_tri_layer(_LOWER, _RAISE, _ADJUST); | |||
} else { | |||
layer_off(_LOWER); | |||
update_tri_layer(_LOWER, _RAISE, _ADJUST); | |||
} | |||
return false; | |||
break; | |||
case RAISE: | |||
if (record->event.pressed) { | |||
layer_on(_RAISE); | |||
update_tri_layer(_LOWER, _RAISE, _ADJUST); | |||
} else { | |||
layer_off(_RAISE); | |||
update_tri_layer(_LOWER, _RAISE, _ADJUST); | |||
} | |||
return false; | |||
break; | |||
case ADJUST: | |||
if (record->event.pressed) { | |||
layer_on(_ADJUST); | |||
} else { | |||
layer_off(_ADJUST); | |||
} | |||
return false; | |||
break; | |||
} | |||
return true; | |||
} |
@ -0,0 +1,22 @@ | |||
# Build Options | |||
# change to "no" to disable the options, or define them in the Makefile in | |||
# the appropriate keymap folder that will get included automatically | |||
# | |||
OLED_ENABLE = no | |||
RGBLIGHT_ENABLE = no | |||
BOOTMAGIC_ENABLE = no # Virtual DIP switch configuration(+1000) | |||
MOUSEKEY_ENABLE = no # Mouse keys(+4700) | |||
EXTRAKEY_ENABLE = no # Audio control and System control(+450) | |||
CONSOLE_ENABLE = no # Console for debug(+400) | |||
COMMAND_ENABLE = no # Commands for debug and configuration | |||
NKRO_ENABLE = no # Nkey Rollover - if this doesn't work, see here: https://github.com/tmk/tmk_keyboard/wiki/FAQ#nkro-doesnt-work | |||
MIDI_ENABLE = no # MIDI controls | |||
AUDIO_ENABLE = no # Audio output on port C6 | |||
UNICODE_ENABLE = no # Unicode | |||
BLUETOOTH_ENABLE = no # Enable Bluetooth with the Adafruit EZ-Key HID | |||
ONEHAND_ENABLE = no # Enable one-hand typing | |||
# Do not enable SLEEP_LED_ENABLE. it uses the same timer as BACKLIGHT_ENABLE | |||
SLEEP_LED_ENABLE = no # Breathing sleep LED during USB suspend |
@ -0,0 +1 @@ | |||
#include "lily58.h" |
@ -0,0 +1,28 @@ | |||
#ifndef LILY58_H | |||
#define LILY58_H | |||
#include "quantum.h" | |||
#ifdef KEYBOARD_lily58_rev1 | |||
#include "rev1.h" | |||
#endif | |||
// Used to create a keymap using only KC_ prefixed keys | |||
#define LAYOUT_kc( \ | |||
L00, L01, L02, L03, L04, L05, R00, R01, R02, R03, R04, R05, \ | |||
L10, L11, L12, L13, L14, L15, R10, R11, R12, R13, R14, R15, \ | |||
L20, L21, L22, L23, L24, L25, R20, R21, R22, R23, R24, R25, \ | |||
L30, L31, L32, L33, L34, L35, L45, R40, R30, R31, R32, R33, R34, R35, \ | |||
L41, L42, L43, L44, R41, R42, R43, R44 \ | |||
) \ | |||
LAYOUT( \ | |||
KC_##L00, KC_##L01, KC_##L02, KC_##L03, KC_##L04, KC_##L05, KC_##R00, KC_##R01, KC_##R02, KC_##R03, KC_##R04, KC_##R05, \ | |||
KC_##L10, KC_##L11, KC_##L12, KC_##L13, KC_##L14, KC_##L15, KC_##R10, KC_##R11, KC_##R12, KC_##R13, KC_##R14, KC_##R15, \ | |||
KC_##L20, KC_##L21, KC_##L22, KC_##L23, KC_##L24, KC_##L25, KC_##R20, KC_##R21, KC_##R22, KC_##R23, KC_##R24, KC_##R25, \ | |||
KC_##L30, KC_##L31, KC_##L32, KC_##L33, KC_##L34, KC_##L35, KC_##L45, KC_##R40, KC_##R30, KC_##R31, KC_##R32, KC_##R33, KC_##R34, KC_##R35, \ | |||
KC_##L41, KC_##L42, KC_##L43, KC_##L44, KC_##R41, KC_##R42, KC_##R43, KC_##R44 \ | |||
) | |||
#endif |
@ -0,0 +1,459 @@ | |||
/* | |||
Copyright 2012 Jun Wako <wakojun@gmail.com> | |||
This program is free software: you can redistribute it and/or modify | |||
it under the terms of the GNU General Public License as published by | |||
the Free Software Foundation, either version 2 of the License, or | |||
(at your option) any later version. | |||
This program is distributed in the hope that it will be useful, | |||
but WITHOUT ANY WARRANTY; without even the implied warranty of | |||
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the | |||
GNU General Public License for more details. | |||
You should have received a copy of the GNU General Public License | |||
along with this program. If not, see <http://www.gnu.org/licenses/>. | |||
*/ | |||
/* | |||
* scan matrix | |||
*/ | |||
#include <stdint.h> | |||
#include <stdbool.h> | |||
#include <avr/io.h> | |||
#include "wait.h" | |||
#include "print.h" | |||
#include "debug.h" | |||
#include "util.h" | |||
#include "matrix.h" | |||
#include "split_util.h" | |||
#include "pro_micro.h" | |||
#include "config.h" | |||
#include "timer.h" | |||
#ifdef USE_I2C | |||
# include "i2c.h" | |||
#else // USE_SERIAL | |||
# include "serial.h" | |||
#endif | |||
#ifndef DEBOUNCING_DELAY | |||
# define DEBOUNCING_DELAY 5 | |||
#endif | |||
#if (DEBOUNCING_DELAY > 0) | |||
static uint16_t debouncing_time; | |||
static bool debouncing = false; | |||
#endif | |||
#if (MATRIX_COLS <= 8) | |||
# define print_matrix_header() print("\nr/c 01234567\n") | |||
# define print_matrix_row(row) print_bin_reverse8(matrix_get_row(row)) | |||
# define matrix_bitpop(i) bitpop(matrix[i]) | |||
# define ROW_SHIFTER ((uint8_t)1) | |||
#else | |||
# error "Currently only supports 8 COLS" | |||
#endif | |||
static matrix_row_t matrix_debouncing[MATRIX_ROWS]; | |||
#define ERROR_DISCONNECT_COUNT 5 | |||
#define ROWS_PER_HAND (MATRIX_ROWS/2) | |||
static uint8_t error_count = 0; | |||
static const uint8_t row_pins[MATRIX_ROWS] = MATRIX_ROW_PINS; | |||
static const uint8_t col_pins[MATRIX_COLS] = MATRIX_COL_PINS; | |||
/* matrix state(1:on, 0:off) */ | |||
static matrix_row_t matrix[MATRIX_ROWS]; | |||
static matrix_row_t matrix_debouncing[MATRIX_ROWS]; | |||
#if (DIODE_DIRECTION == COL2ROW) | |||
static void init_cols(void); | |||
static bool read_cols_on_row(matrix_row_t current_matrix[], uint8_t current_row); | |||
static void unselect_rows(void); | |||
static void select_row(uint8_t row); | |||
static void unselect_row(uint8_t row); | |||
#elif (DIODE_DIRECTION == ROW2COL) | |||
static void init_rows(void); | |||
static bool read_rows_on_col(matrix_row_t current_matrix[], uint8_t current_col); | |||
static void unselect_cols(void); | |||
static void unselect_col(uint8_t col); | |||
static void select_col(uint8_t col); | |||
#endif | |||
__attribute__ ((weak)) | |||
void matrix_init_kb(void) { | |||
matrix_init_user(); | |||
} | |||
__attribute__ ((weak)) | |||
void matrix_scan_kb(void) { | |||
matrix_scan_user(); | |||
} | |||
__attribute__ ((weak)) | |||
void matrix_init_user(void) { | |||
} | |||
__attribute__ ((weak)) | |||
void matrix_scan_user(void) { | |||
} | |||
inline | |||
uint8_t matrix_rows(void) | |||
{ | |||
return MATRIX_ROWS; | |||
} | |||
inline | |||
uint8_t matrix_cols(void) | |||
{ | |||
return MATRIX_COLS; | |||
} | |||
void matrix_init(void) | |||
{ | |||
debug_enable = true; | |||
debug_matrix = true; | |||
debug_mouse = true; | |||
// initialize row and col | |||
#if (DIODE_DIRECTION == COL2ROW) | |||
unselect_rows(); | |||
init_cols(); | |||
#elif (DIODE_DIRECTION == ROW2COL) | |||
unselect_cols(); | |||
init_rows(); | |||
#endif | |||
TX_RX_LED_INIT; | |||
// initialize matrix state: all keys off | |||
for (uint8_t i=0; i < MATRIX_ROWS; i++) { | |||
matrix[i] = 0; | |||
matrix_debouncing[i] = 0; | |||
} | |||
matrix_init_quantum(); | |||
} | |||
uint8_t _matrix_scan(void) | |||
{ | |||
int offset = isLeftHand ? 0 : (ROWS_PER_HAND); | |||
#if (DIODE_DIRECTION == COL2ROW) | |||
// Set row, read cols | |||
for (uint8_t current_row = 0; current_row < ROWS_PER_HAND; current_row++) { | |||
# if (DEBOUNCING_DELAY > 0) | |||
bool matrix_changed = read_cols_on_row(matrix_debouncing+offset, current_row); | |||
if (matrix_changed) { | |||
debouncing = true; | |||
debouncing_time = timer_read(); | |||
} | |||
# else | |||
read_cols_on_row(matrix+offset, current_row); | |||
# endif | |||
} | |||
#elif (DIODE_DIRECTION == ROW2COL) | |||
// Set col, read rows | |||
for (uint8_t current_col = 0; current_col < MATRIX_COLS; current_col++) { | |||
# if (DEBOUNCING_DELAY > 0) | |||
bool matrix_changed = read_rows_on_col(matrix_debouncing+offset, current_col); | |||
if (matrix_changed) { | |||
debouncing = true; | |||
debouncing_time = timer_read(); | |||
} | |||
# else | |||
read_rows_on_col(matrix+offset, current_col); | |||
# endif | |||
} | |||
#endif | |||
# if (DEBOUNCING_DELAY > 0) | |||
if (debouncing && (timer_elapsed(debouncing_time) > DEBOUNCING_DELAY)) { | |||
for (uint8_t i = 0; i < ROWS_PER_HAND; i++) { | |||
matrix[i+offset] = matrix_debouncing[i+offset]; | |||
} | |||
debouncing = false; | |||
} | |||
# endif | |||
return 1; | |||
} | |||
#ifdef USE_I2C | |||
// Get rows from other half over i2c | |||
int i2c_transaction(void) { | |||
int slaveOffset = (isLeftHand) ? (ROWS_PER_HAND) : 0; | |||
int err = i2c_master_start(SLAVE_I2C_ADDRESS + I2C_WRITE); | |||
if (err) goto i2c_error; | |||
// start of matrix stored at 0x00 | |||
err = i2c_master_write(0x00); | |||
if (err) goto i2c_error; | |||
// Start read | |||
err = i2c_master_start(SLAVE_I2C_ADDRESS + I2C_READ); | |||
if (err) goto i2c_error; | |||
if (!err) { | |||
int i; | |||
for (i = 0; i < ROWS_PER_HAND-1; ++i) { | |||
matrix[slaveOffset+i] = i2c_master_read(I2C_ACK); | |||
} | |||
matrix[slaveOffset+i] = i2c_master_read(I2C_NACK); | |||
i2c_master_stop(); | |||
} else { | |||
i2c_error: // the cable is disconnceted, or something else went wrong | |||
i2c_reset_state(); | |||
return err; | |||
} | |||
return 0; | |||
} | |||
#else // USE_SERIAL | |||
int serial_transaction(void) { | |||
int slaveOffset = (isLeftHand) ? (ROWS_PER_HAND) : 0; | |||
if (serial_update_buffers()) { | |||
return 1; | |||
} | |||
for (int i = 0; i < ROWS_PER_HAND; ++i) { | |||
matrix[slaveOffset+i] = serial_slave_buffer[i]; | |||
} | |||
return 0; | |||
} | |||
#endif | |||
uint8_t matrix_scan(void) | |||
{ | |||
uint8_t ret = _matrix_scan(); | |||
#ifdef USE_I2C | |||
if( i2c_transaction() ) { | |||
#else // USE_SERIAL | |||
if( serial_transaction() ) { | |||
#endif | |||
// turn on the indicator led when halves are disconnected | |||
TXLED1; | |||
error_count++; | |||
if (error_count > ERROR_DISCONNECT_COUNT) { | |||
// reset other half if disconnected | |||
int slaveOffset = (isLeftHand) ? (ROWS_PER_HAND) : 0; | |||
for (int i = 0; i < ROWS_PER_HAND; ++i) { | |||
matrix[slaveOffset+i] = 0; | |||
} | |||
} | |||
} else { | |||
// turn off the indicator led on no error | |||
TXLED0; | |||
error_count = 0; | |||
} | |||
matrix_scan_quantum(); | |||
return ret; | |||
} | |||
void matrix_slave_scan(void) { | |||
_matrix_scan(); | |||
int offset = (isLeftHand) ? 0 : ROWS_PER_HAND; | |||
#ifdef USE_I2C | |||
for (int i = 0; i < ROWS_PER_HAND; ++i) { | |||
i2c_slave_buffer[i] = matrix[offset+i]; | |||
} | |||
#else // USE_SERIAL | |||
for (int i = 0; i < ROWS_PER_HAND; ++i) { | |||
serial_slave_buffer[i] = matrix[offset+i]; | |||
} | |||
#endif | |||
} | |||
bool matrix_is_modified(void) | |||
{ | |||
if (debouncing) return false; | |||
return true; | |||
} | |||
inline | |||
bool matrix_is_on(uint8_t row, uint8_t col) | |||
{ | |||
return (matrix[row] & ((matrix_row_t)1<<col)); | |||
} | |||
inline | |||
matrix_row_t matrix_get_row(uint8_t row) | |||
{ | |||
return matrix[row]; | |||
} | |||
void matrix_print(void) | |||
{ | |||
print("\nr/c 0123456789ABCDEF\n"); | |||
for (uint8_t row = 0; row < MATRIX_ROWS; row++) { | |||
phex(row); print(": "); | |||
pbin_reverse16(matrix_get_row(row)); | |||
print("\n"); | |||
} | |||
} | |||
uint8_t matrix_key_count(void) | |||
{ | |||
uint8_t count = 0; | |||
for (uint8_t i = 0; i < MATRIX_ROWS; i++) { | |||
count += bitpop16(matrix[i]); | |||
} | |||
return count; | |||
} | |||
#if (DIODE_DIRECTION == COL2ROW) | |||
static void init_cols(void) | |||
{ | |||
for(uint8_t x = 0; x < MATRIX_COLS; x++) { | |||
uint8_t pin = col_pins[x]; | |||
_SFR_IO8((pin >> 4) + 1) &= ~_BV(pin & 0xF); // IN | |||
_SFR_IO8((pin >> 4) + 2) |= _BV(pin & 0xF); // HI | |||
} | |||
} | |||
static bool read_cols_on_row(matrix_row_t current_matrix[], uint8_t current_row) | |||
{ | |||
// Store last value of row prior to reading | |||
matrix_row_t last_row_value = current_matrix[current_row]; | |||
// Clear data in matrix row | |||
current_matrix[current_row] = 0; | |||
// Select row and wait for row selecton to stabilize | |||
select_row(current_row); | |||
wait_us(30); | |||
// For each col... | |||
for(uint8_t col_index = 0; col_index < MATRIX_COLS; col_index++) { | |||
// Select the col pin to read (active low) | |||
uint8_t pin = col_pins[col_index]; | |||
uint8_t pin_state = (_SFR_IO8(pin >> 4) & _BV(pin & 0xF)); | |||
// Populate the matrix row with the state of the col pin | |||
current_matrix[current_row] |= pin_state ? 0 : (ROW_SHIFTER << col_index); | |||
} | |||
// Unselect row | |||
unselect_row(current_row); | |||
return (last_row_value != current_matrix[current_row]); | |||
} | |||
static void select_row(uint8_t row) | |||
{ | |||
uint8_t pin = row_pins[row]; | |||
_SFR_IO8((pin >> 4) + 1) |= _BV(pin & 0xF); // OUT | |||
_SFR_IO8((pin >> 4) + 2) &= ~_BV(pin & 0xF); // LOW | |||
} | |||
static void unselect_row(uint8_t row) | |||
{ | |||
uint8_t pin = row_pins[row]; | |||
_SFR_IO8((pin >> 4) + 1) &= ~_BV(pin & 0xF); // IN | |||
_SFR_IO8((pin >> 4) + 2) |= _BV(pin & 0xF); // HI | |||
} | |||
static void unselect_rows(void) | |||
{ | |||
for(uint8_t x = 0; x < ROWS_PER_HAND; x++) { | |||
uint8_t pin = row_pins[x]; | |||
_SFR_IO8((pin >> 4) + 1) &= ~_BV(pin & 0xF); // IN | |||
_SFR_IO8((pin >> 4) + 2) |= _BV(pin & 0xF); // HI | |||
} | |||
} | |||
#elif (DIODE_DIRECTION == ROW2COL) | |||
static void init_rows(void) | |||
{ | |||
for(uint8_t x = 0; x < ROWS_PER_HAND; x++) { | |||
uint8_t pin = row_pins[x]; | |||
_SFR_IO8((pin >> 4) + 1) &= ~_BV(pin & 0xF); // IN | |||
_SFR_IO8((pin >> 4) + 2) |= _BV(pin & 0xF); // HI | |||
} | |||
} | |||
static bool read_rows_on_col(matrix_row_t current_matrix[], uint8_t current_col) | |||
{ | |||
bool matrix_changed = false; | |||
// Select col and wait for col selecton to stabilize | |||
select_col(current_col); | |||
wait_us(30); | |||
// For each row... | |||
for(uint8_t row_index = 0; row_index < ROWS_PER_HAND; row_index++) | |||
{ | |||
// Store last value of row prior to reading | |||
matrix_row_t last_row_value = current_matrix[row_index]; | |||
// Check row pin state | |||
if ((_SFR_IO8(row_pins[row_index] >> 4) & _BV(row_pins[row_index] & 0xF)) == 0) | |||
{ | |||
// Pin LO, set col bit | |||
current_matrix[row_index] |= (ROW_SHIFTER << current_col); | |||
} | |||
else | |||
{ | |||
// Pin HI, clear col bit | |||
current_matrix[row_index] &= ~(ROW_SHIFTER << current_col); | |||
} | |||
// Determine if the matrix changed state | |||
if ((last_row_value != current_matrix[row_index]) && !(matrix_changed)) | |||
{ | |||
matrix_changed = true; | |||
} | |||
} | |||
// Unselect col | |||
unselect_col(current_col); | |||
return matrix_changed; | |||
} | |||
static void select_col(uint8_t col) | |||
{ | |||
uint8_t pin = col_pins[col]; | |||
_SFR_IO8((pin >> 4) + 1) |= _BV(pin & 0xF); // OUT | |||
_SFR_IO8((pin >> 4) + 2) &= ~_BV(pin & 0xF); // LOW | |||
} | |||
static void unselect_col(uint8_t col) | |||
{ | |||
uint8_t pin = col_pins[col]; | |||
_SFR_IO8((pin >> 4) + 1) &= ~_BV(pin & 0xF); // IN | |||
_SFR_IO8((pin >> 4) + 2) |= _BV(pin & 0xF); // HI | |||
} | |||
static void unselect_cols(void) | |||
{ | |||
for(uint8_t x = 0; x < MATRIX_COLS; x++) { | |||
uint8_t pin = col_pins[x]; | |||
_SFR_IO8((pin >> 4) + 1) &= ~_BV(pin & 0xF); // IN | |||
_SFR_IO8((pin >> 4) + 2) |= _BV(pin & 0xF); // HI | |||
} | |||
} | |||
#endif |
@ -0,0 +1,17 @@ | |||
# Lily58 | |||
Lily58 is 6×4+5keys column-staggered split keyboard. | |||
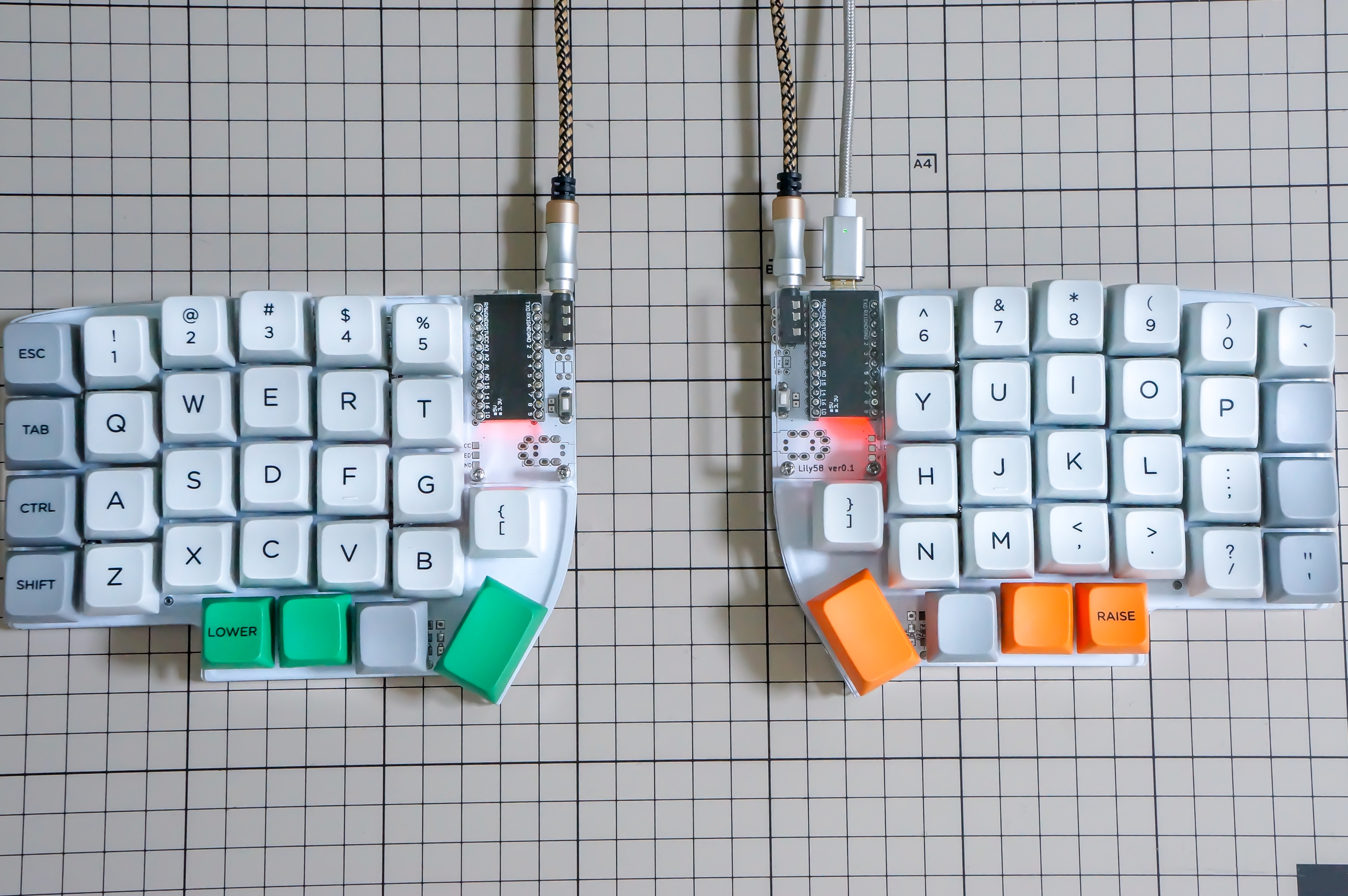 | |||
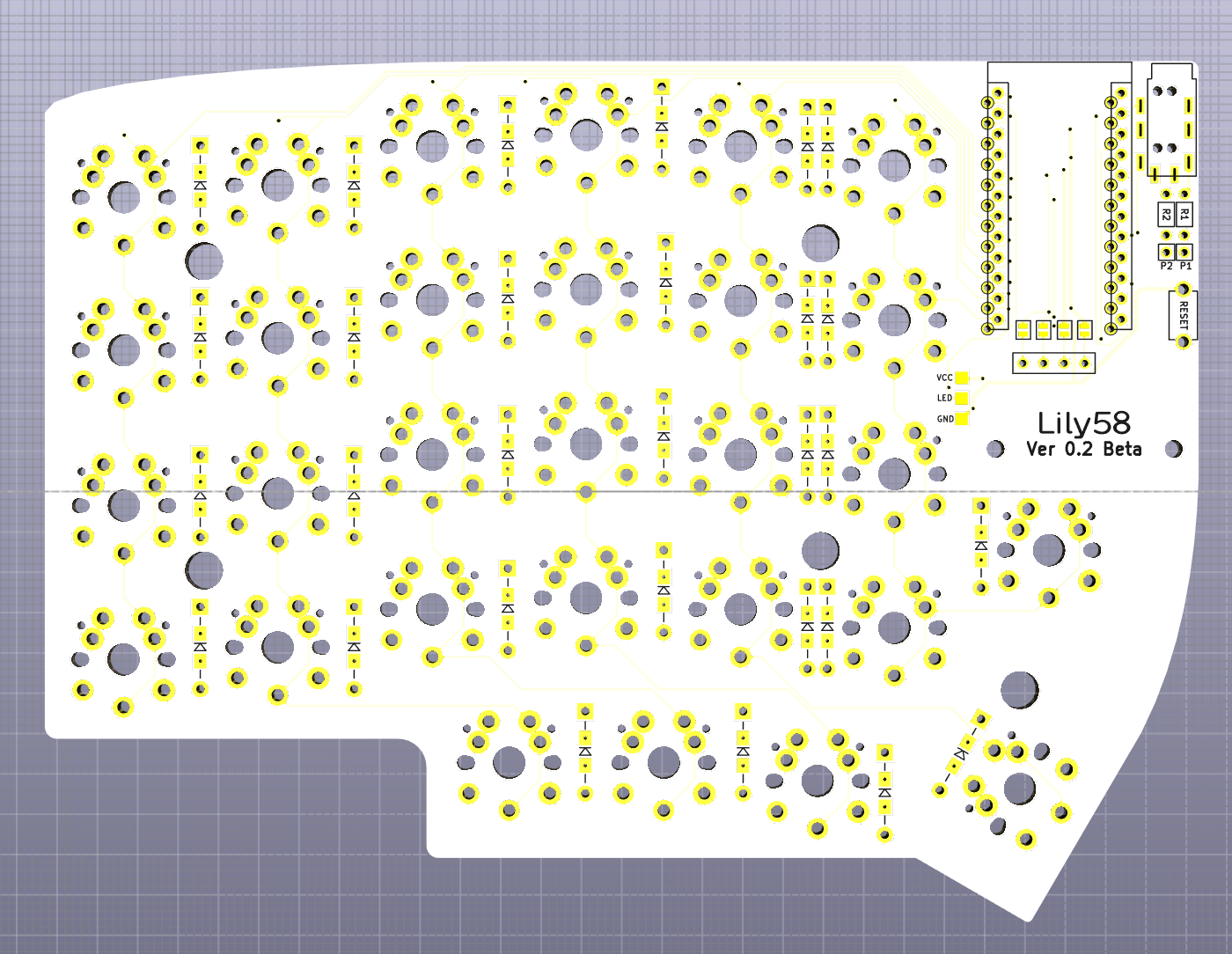 | |||
Keyboard Maintainer: [Naoki Katahira](https://github.com/kata0510/) [Twitter:@F_YUUCHI](https://twitter.com/F_YUUCHI) | |||
Hardware Supported: Lily58 PCB, ProMicro | |||
Hardware Availability: [PCB & Case Data](https://github.com/kata0510/Lily58) | |||
Make example for this keyboard (after setting up your build environment): | |||
make lily58:default | |||
See the [build environment setup](https://docs.qmk.fm/#/getting_started_build_tools) and the [make instructions](https://docs.qmk.fm/#/getting_started_make_guide) for more information. Brand new to QMK? Start with our [Complete Newbs Guide](https://docs.qmk.fm/#/newbs). |
@ -0,0 +1,86 @@ | |||
/* | |||
Copyright 2012 Jun Wako <wakojun@gmail.com> | |||
Copyright 2015 Jack Humbert | |||
Copyright 2017 F_YUUCHI | |||
This program is free software: you can redistribute it and/or modify | |||
it under the terms of the GNU General Public License as published by | |||
the Free Software Foundation, either version 2 of the License, or | |||
(at your option) any later version. | |||
This program is distributed in the hope that it will be useful, | |||
but WITHOUT ANY WARRANTY; without even the implied warranty of | |||
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the | |||
GNU General Public License for more details. | |||
You should have received a copy of the GNU General Public License | |||
along with this program. If not, see <http://www.gnu.org/licenses/>. | |||
*/ | |||
#pragma once | |||
//#include QMK_KEYBOARD_CONFIG_H | |||
#include "config_common.h" | |||
/* USB Device descriptor parameter */ | |||
#define VENDOR_ID 0xFC51 | |||
#define PRODUCT_ID 0x0058 | |||
#define DEVICE_VER 0x0100 | |||
#define MANUFACTURER F_YUUCHI | |||
#define PRODUCT Lily58 | |||
#define DESCRIPTION Lily58 is 6×4+5keys column-staggered split keyboard. | |||
/* key matrix size */ | |||
// Rows are doubled-up | |||
#define MATRIX_ROWS 10 | |||
#define MATRIX_COLS 6 | |||
// wiring of each half | |||
#define MATRIX_ROW_PINS { C6, D7, E6, B4, B5 } | |||
#define MATRIX_COL_PINS { F6, F7, B1, B3, B2, B6 } | |||
#define CATERINA_BOOTLOADER | |||
/* define tapping term */ | |||
#define TAPPING_TERM 100 | |||
/* define if matrix has ghost */ | |||
//#define MATRIX_HAS_GHOST | |||
/* Set 0 if debouncing isn't needed */ | |||
#define DEBOUNCING_DELAY 5 | |||
/* Mechanical locking support. Use KC_LCAP, KC_LNUM or KC_LSCR instead in keymap */ | |||
#define LOCKING_SUPPORT_ENABLE | |||
/* Locking resynchronize hack */ | |||
#define LOCKING_RESYNC_ENABLE | |||
/* key combination for command */ | |||
#define IS_COMMAND() ( \ | |||
keyboard_report->mods == (MOD_BIT(KC_LSHIFT) | MOD_BIT(KC_RSHIFT)) \ | |||
) | |||
/* ws2812 RGB LED */ | |||
#define RGB_DI_PIN D3 | |||
#define RGBLIGHT_TIMER | |||
#define RGBLED_NUM 14 // Number of LEDs | |||
#define ws2812_PORTREG PORTD | |||
#define ws2812_DDRREG DDRD | |||
/* | |||
* Feature disable options | |||
* These options are also useful to firmware size reduction. | |||
*/ | |||
/* disable debug print */ | |||
// #define NO_DEBUG | |||
/* disable print */ | |||
// #define NO_PRINT | |||
/* disable action features */ | |||
//#define NO_ACTION_LAYER | |||
//#define NO_ACTION_TAPPING | |||
//#define NO_ACTION_ONESHOT | |||
//#define NO_ACTION_MACRO | |||
//#define NO_ACTION_FUNCTION |
@ -0,0 +1,24 @@ | |||
#include "lily58.h" | |||
/* | |||
#ifdef SSD1306OLED | |||
void led_set_kb(uint8_t usb_led) { | |||
// put your keyboard LED indicator (ex: Caps Lock LED) toggling code here | |||
led_set_user(usb_led); | |||
} | |||
#endif | |||
*/ | |||
void matrix_init_kb(void) { | |||
// // green led on | |||
// DDRD |= (1<<5); | |||
// PORTD &= ~(1<<5); | |||
// // orange led on | |||
// DDRB |= (1<<0); | |||
// PORTB &= ~(1<<0); | |||
matrix_init_user(); | |||
}; | |||
@ -0,0 +1,61 @@ | |||
#pragma once | |||
#include "lily58.h" | |||
//void promicro_bootloader_jmp(bool program); | |||
#include "quantum.h" | |||
#ifdef USE_I2C | |||
#include <stddef.h> | |||
#ifdef __AVR__ | |||
#include <avr/io.h> | |||
#include <avr/interrupt.h> | |||
#endif | |||
#endif | |||
//void promicro_bootloader_jmp(bool program); | |||
#ifndef FLIP_HALF | |||
#define LAYOUT( \ | |||
L00, L01, L02, L03, L04, L05, R00, R01, R02, R03, R04, R05, \ | |||
L10, L11, L12, L13, L14, L15, R10, R11, R12, R13, R14, R15, \ | |||
L20, L21, L22, L23, L24, L25, R20, R21, R22, R23, R24, R25, \ | |||
L30, L31, L32, L33, L34, L35, L45, R40, R30, R31, R32, R33, R34, R35, \ | |||
L41, L42, L43, L44, R41, R42, R43, R44 \ | |||
) \ | |||
{ \ | |||
{ L00, L01, L02, L03, L04, L05 }, \ | |||
{ L10, L11, L12, L13, L14, L15 }, \ | |||
{ L20, L21, L22, L23, L24, L25 }, \ | |||
{ L30, L31, L32, L33, L34, L35 }, \ | |||
{ KC_NO, L41, L42, L43, L44, L45 }, \ | |||
{ R05, R04, R03, R02, R01, R00 }, \ | |||
{ R15, R14, R13, R12, R11, R10 }, \ | |||
{ R25, R24, R23, R22, R21, R20 }, \ | |||
{ R35, R34, R33, R32, R31, R30 }, \ | |||
{ KC_NO, R44, R43, R42, R41, R40 } \ | |||
} | |||
#else | |||
// Keymap with right side flipped | |||
// (TRRS jack on both halves are to the right) | |||
#define LAYOUT( \ | |||
L00, L01, L02, L03, L04, L05, R00, R01, R02, R03, R04, R05, \ | |||
L10, L11, L12, L13, L14, L15, R10, R11, R12, R13, R14, R15, \ | |||
L20, L21, L22, L23, L24, L25, R20, R21, R22, R23, R24, R25, \ | |||
L30, L31, L32, L33, L34, L35, L45, R30, R31, R32, R33, R34, R35, R45, \ | |||
L41, L42, L43, L44, R41, R42, R43, R44 \ | |||
) \ | |||
{ \ | |||
{ L00, L01, L02, L03, L04, L05 }, \ | |||
{ L10, L11, L12, L13, L14, L15 }, \ | |||
{ L20, L21, L22, L23, L24, L25 }, \ | |||
{ L30, L31, L32, L33, L34, L35 }, \ | |||
{ KC_NO, L41, L42, L43, L44, L45 }, \ | |||
{ R00, R01, R02, R03, R04, R05 }, \ | |||
{ R10, R11, R12, R13, R14, R15 }, \ | |||
{ R20, R21, R22, R23, R24, R25 }, \ | |||
{ R30, R31, R32, R33, R34, R35 }, \ | |||
{ KC_NO, R41, R42, R43, R44, R45 } \ | |||
} | |||
#endif |
@ -0,0 +1 @@ | |||
BACKLIGHT_ENABLE = no |
@ -0,0 +1,76 @@ | |||
SRC += matrix.c \ | |||
i2c.c \ | |||
split_util.c \ | |||
serial.c \ | |||
ssd1306.c | |||
# MCU name | |||
#MCU = at90usb1287 | |||
MCU = atmega32u4 | |||
# Processor frequency. | |||
# This will define a symbol, F_CPU, in all source code files equal to the | |||
# processor frequency in Hz. You can then use this symbol in your source code to | |||
# calculate timings. Do NOT tack on a 'UL' at the end, this will be done | |||
# automatically to create a 32-bit value in your source code. | |||
# | |||
# This will be an integer division of F_USB below, as it is sourced by | |||
# F_USB after it has run through any CPU prescalers. Note that this value | |||
# does not *change* the processor frequency - it should merely be updated to | |||
# reflect the processor speed set externally so that the code can use accurate | |||
# software delays. | |||
F_CPU = 16000000 | |||
# | |||
# LUFA specific | |||
# | |||
# Target architecture (see library "Board Types" documentation). | |||
ARCH = AVR8 | |||
# Input clock frequency. | |||
# This will define a symbol, F_USB, in all source code files equal to the | |||
# input clock frequency (before any prescaling is performed) in Hz. This value may | |||
# differ from F_CPU if prescaling is used on the latter, and is required as the | |||
# raw input clock is fed directly to the PLL sections of the AVR for high speed | |||
# clock generation for the USB and other AVR subsections. Do NOT tack on a 'UL' | |||
# at the end, this will be done automatically to create a 32-bit value in your | |||
# source code. | |||
# | |||
# If no clock division is performed on the input clock inside the AVR (via the | |||
# CPU clock adjust registers or the clock division fuses), this will be equal to F_CPU. | |||
F_USB = $(F_CPU) | |||
# Interrupt driven control endpoint task(+60) | |||
OPT_DEFS += -DINTERRUPT_CONTROL_ENDPOINT | |||
# Bootloader | |||
# This definition is optional, and if your keyboard supports multiple bootloaders of | |||
# different sizes, comment this out, and the correct address will be loaded | |||
# automatically (+60). See bootloader.mk for all options. | |||
BOOTLOADER = caterina | |||
# Build Options | |||
# change to "no" to disable the options, or define them in the Makefile in | |||
# the appropriate keymap folder that will get included automatically | |||
# | |||
BOOTMAGIC_ENABLE = no # Virtual DIP switch configuration(+1000) | |||
MOUSEKEY_ENABLE = no # Mouse keys(+4700) | |||
EXTRAKEY_ENABLE = no # Audio control and System control(+450) | |||
CONSOLE_ENABLE = no # Console for debug(+400) | |||
COMMAND_ENABLE = no # Commands for debug and configuration | |||
NKRO_ENABLE = no # Nkey Rollover - if this doesn't work, see here: https://github.com/tmk/tmk_keyboard/wiki/FAQ#nkro-doesnt-work | |||
BACKLIGHT_ENABLE = no # Enable keyboard backlight functionality | |||
MIDI_ENABLE = no # MIDI controls | |||
AUDIO_ENABLE = no # Audio output on port C6 | |||
UNICODE_ENABLE = no # Unicode | |||
BLUETOOTH_ENABLE = no # Enable Bluetooth with the Adafruit EZ-Key HID | |||
RGBLIGHT_ENABLE = no # Enable WS2812 RGB underlight. Do not enable this with audio at the same time. | |||
SUBPROJECT_rev1 = no | |||
USE_I2C = no | |||
# Do not enable SLEEP_LED_ENABLE. it uses the same timer as BACKLIGHT_ENABLE | |||
SLEEP_LED_ENABLE = no # Breathing sleep LED during USB suspend | |||
CUSTOM_MATRIX = yes | |||
DEFAULT_FOLDER = lily58/rev1 |
@ -0,0 +1,445 @@ | |||
/* | |||
* WARNING: be careful changing this code, it is very timing dependent | |||
*/ | |||
#ifndef F_CPU | |||
#define F_CPU 16000000 | |||
#endif | |||
#include <avr/io.h> | |||
#include <avr/interrupt.h> | |||
#include <util/delay.h> | |||
#include <stddef.h> | |||
#include <stdbool.h> | |||
#include "serial.h" | |||
//#include <pro_micro.h> | |||
#ifdef USE_SERIAL | |||
//#ifndef USE_SERIAL_PD2 | |||
#ifndef SERIAL_USE_MULTI_TRANSACTION | |||
/* --- USE Simple API (OLD API, compatible with let's split serial.c) */ | |||
#if SERIAL_SLAVE_BUFFER_LENGTH > 0 | |||
uint8_t volatile serial_slave_buffer[SERIAL_SLAVE_BUFFER_LENGTH] = {0}; | |||
#endif | |||
#if SERIAL_MASTER_BUFFER_LENGTH > 0 | |||
uint8_t volatile serial_master_buffer[SERIAL_MASTER_BUFFER_LENGTH] = {0}; | |||
#endif | |||
uint8_t volatile status0 = 0; | |||
SSTD_t transactions[] = { | |||
{ (uint8_t *)&status0, | |||
#if SERIAL_MASTER_BUFFER_LENGTH > 0 | |||
sizeof(serial_master_buffer), (uint8_t *)serial_master_buffer, | |||
#else | |||
0, (uint8_t *)NULL, | |||
#endif | |||
#if SERIAL_SLAVE_BUFFER_LENGTH > 0 | |||
sizeof(serial_slave_buffer), (uint8_t *)serial_slave_buffer | |||
#else | |||
0, (uint8_t *)NULL, | |||
#endif | |||
} | |||
}; | |||
void serial_master_init(void) | |||
{ soft_serial_initiator_init(transactions); } | |||
void serial_slave_init(void) | |||
{ soft_serial_target_init(transactions); } | |||
// 0 => no error | |||
// 1 => slave did not respond | |||
// 2 => checksum error | |||
int serial_update_buffers() | |||
{ return soft_serial_transaction(); } | |||
#endif // Simple API (OLD API, compatible with let's split serial.c) | |||
#define ALWAYS_INLINE __attribute__((always_inline)) | |||
#define NO_INLINE __attribute__((noinline)) | |||
#define _delay_sub_us(x) __builtin_avr_delay_cycles(x) | |||
// Serial pulse period in microseconds. | |||
#define TID_SEND_ADJUST 14 | |||
#define SELECT_SERIAL_SPEED 1 | |||
#if SELECT_SERIAL_SPEED == 0 | |||
// Very High speed | |||
#define SERIAL_DELAY 4 // micro sec | |||
#define READ_WRITE_START_ADJUST 33 // cycles | |||
#define READ_WRITE_WIDTH_ADJUST 3 // cycles | |||
#elif SELECT_SERIAL_SPEED == 1 | |||
// High speed | |||
#define SERIAL_DELAY 6 // micro sec | |||
#define READ_WRITE_START_ADJUST 30 // cycles | |||
#define READ_WRITE_WIDTH_ADJUST 3 // cycles | |||
#elif SELECT_SERIAL_SPEED == 2 | |||
// Middle speed | |||
#define SERIAL_DELAY 12 // micro sec | |||
#define READ_WRITE_START_ADJUST 30 // cycles | |||
#define READ_WRITE_WIDTH_ADJUST 3 // cycles | |||
#elif SELECT_SERIAL_SPEED == 3 | |||
// Low speed | |||
#define SERIAL_DELAY 24 // micro sec | |||
#define READ_WRITE_START_ADJUST 30 // cycles | |||
#define READ_WRITE_WIDTH_ADJUST 3 // cycles | |||
#elif SELECT_SERIAL_SPEED == 4 | |||
// Very Low speed | |||
#define SERIAL_DELAY 50 // micro sec | |||
#define READ_WRITE_START_ADJUST 30 // cycles | |||
#define READ_WRITE_WIDTH_ADJUST 3 // cycles | |||
#else | |||
#error Illegal Serial Speed | |||
#endif | |||
#define SERIAL_DELAY_HALF1 (SERIAL_DELAY/2) | |||
#define SERIAL_DELAY_HALF2 (SERIAL_DELAY - SERIAL_DELAY/2) | |||
#define SLAVE_INT_WIDTH_US 1 | |||
#ifndef SERIAL_USE_MULTI_TRANSACTION | |||
#define SLAVE_INT_RESPONSE_TIME SERIAL_DELAY | |||
#else | |||
#define SLAVE_INT_ACK_WIDTH_UNIT 2 | |||
#define SLAVE_INT_ACK_WIDTH 4 | |||
#endif | |||
static SSTD_t *Transaction_table = NULL; | |||
inline static | |||
void serial_delay(void) { | |||
_delay_us(SERIAL_DELAY); | |||
} | |||
inline static | |||
void serial_delay_half1(void) { | |||
_delay_us(SERIAL_DELAY_HALF1); | |||
} | |||
inline static | |||
void serial_delay_half2(void) { | |||
_delay_us(SERIAL_DELAY_HALF2); | |||
} | |||
inline static void serial_output(void) ALWAYS_INLINE; | |||
inline static | |||
void serial_output(void) { | |||
SERIAL_PIN_DDR |= SERIAL_PIN_MASK; | |||
} | |||
// make the serial pin an input with pull-up resistor | |||
inline static void serial_input_with_pullup(void) ALWAYS_INLINE; | |||
inline static | |||
void serial_input_with_pullup(void) { | |||
SERIAL_PIN_DDR &= ~SERIAL_PIN_MASK; | |||
SERIAL_PIN_PORT |= SERIAL_PIN_MASK; | |||
} | |||
inline static | |||
uint8_t serial_read_pin(void) { | |||
return !!(SERIAL_PIN_INPUT & SERIAL_PIN_MASK); | |||
} | |||
inline static void serial_low(void) ALWAYS_INLINE; | |||
inline static | |||
void serial_low(void) { | |||
SERIAL_PIN_PORT &= ~SERIAL_PIN_MASK; | |||
} | |||
inline static void serial_high(void) ALWAYS_INLINE; | |||
inline static | |||
void serial_high(void) { | |||
SERIAL_PIN_PORT |= SERIAL_PIN_MASK; | |||
} | |||
void soft_serial_initiator_init(SSTD_t *sstd_table) | |||
{ | |||
Transaction_table = sstd_table; | |||
serial_output(); | |||
serial_high(); | |||
} | |||
void soft_serial_target_init(SSTD_t *sstd_table) | |||
{ | |||
Transaction_table = sstd_table; | |||
serial_input_with_pullup(); | |||
#if SERIAL_PIN_MASK == _BV(PD0) | |||
// Enable INT0 | |||
EIMSK |= _BV(INT0); | |||
// Trigger on falling edge of INT0 | |||
EICRA &= ~(_BV(ISC00) | _BV(ISC01)); | |||
#elif SERIAL_PIN_MASK == _BV(PD2) | |||
// Enable INT2 | |||
EIMSK |= _BV(INT2); | |||
// Trigger on falling edge of INT2 | |||
EICRA &= ~(_BV(ISC20) | _BV(ISC21)); | |||
#else | |||
#error unknown SERIAL_PIN_MASK value | |||
#endif | |||
} | |||
// Used by the sender to synchronize timing with the reciver. | |||
static void sync_recv(void) NO_INLINE; | |||
static | |||
void sync_recv(void) { | |||
for (uint8_t i = 0; i < SERIAL_DELAY*5 && serial_read_pin(); i++ ) { | |||
} | |||
// This shouldn't hang if the target disconnects because the | |||
// serial line will float to high if the target does disconnect. | |||
while (!serial_read_pin()); | |||
} | |||
// Used by the reciver to send a synchronization signal to the sender. | |||
static void sync_send(void)NO_INLINE; | |||
static | |||
void sync_send(void) { | |||
serial_low(); | |||
serial_delay(); | |||
serial_high(); | |||
} | |||
// Reads a byte from the serial line | |||
static uint8_t serial_read_chunk(uint8_t *pterrcount, uint8_t bit) NO_INLINE; | |||
static uint8_t serial_read_chunk(uint8_t *pterrcount, uint8_t bit) { | |||
uint8_t byte, i, p, pb; | |||
_delay_sub_us(READ_WRITE_START_ADJUST); | |||
for( i = 0, byte = 0, p = 0; i < bit; i++ ) { | |||
serial_delay_half1(); // read the middle of pulses | |||
if( serial_read_pin() ) { | |||
byte = (byte << 1) | 1; p ^= 1; | |||
} else { | |||
byte = (byte << 1) | 0; p ^= 0; | |||
} | |||
_delay_sub_us(READ_WRITE_WIDTH_ADJUST); | |||
serial_delay_half2(); | |||
} | |||
/* recive parity bit */ | |||
serial_delay_half1(); // read the middle of pulses | |||
pb = serial_read_pin(); | |||
_delay_sub_us(READ_WRITE_WIDTH_ADJUST); | |||
serial_delay_half2(); | |||
*pterrcount += (p != pb)? 1 : 0; | |||
return byte; | |||
} | |||
// Sends a byte with MSB ordering | |||
void serial_write_chunk(uint8_t data, uint8_t bit) NO_INLINE; | |||
void serial_write_chunk(uint8_t data, uint8_t bit) { | |||
uint8_t b, p; | |||
for( p = 0, b = 1<<(bit-1); b ; b >>= 1) { | |||
if(data & b) { | |||
serial_high(); p ^= 1; | |||
} else { | |||
serial_low(); p ^= 0; | |||
} | |||
serial_delay(); | |||
} | |||
/* send parity bit */ | |||
if(p & 1) { serial_high(); } | |||
else { serial_low(); } | |||
serial_delay(); | |||
serial_low(); // sync_send() / senc_recv() need raise edge | |||
} | |||
static void serial_send_packet(uint8_t *buffer, uint8_t size) NO_INLINE; | |||
static | |||
void serial_send_packet(uint8_t *buffer, uint8_t size) { | |||
for (uint8_t i = 0; i < size; ++i) { | |||
uint8_t data; | |||
data = buffer[i]; | |||
sync_send(); | |||
serial_write_chunk(data,8); | |||
} | |||
} | |||
static uint8_t serial_recive_packet(uint8_t *buffer, uint8_t size) NO_INLINE; | |||
static | |||
uint8_t serial_recive_packet(uint8_t *buffer, uint8_t size) { | |||
uint8_t pecount = 0; | |||
for (uint8_t i = 0; i < size; ++i) { | |||
uint8_t data; | |||
sync_recv(); | |||
data = serial_read_chunk(&pecount, 8); | |||
buffer[i] = data; | |||
} | |||
return pecount == 0; | |||
} | |||
inline static | |||
void change_sender2reciver(void) { | |||
sync_send(); //0 | |||
serial_delay_half1(); //1 | |||
serial_low(); //2 | |||
serial_input_with_pullup(); //2 | |||
serial_delay_half1(); //3 | |||
} | |||
inline static | |||
void change_reciver2sender(void) { | |||
sync_recv(); //0 | |||
serial_delay(); //1 | |||
serial_low(); //3 | |||
serial_output(); //3 | |||
serial_delay_half1(); //4 | |||
} | |||
// interrupt handle to be used by the target device | |||
ISR(SERIAL_PIN_INTERRUPT) { | |||
#ifndef SERIAL_USE_MULTI_TRANSACTION | |||
serial_low(); | |||
serial_output(); | |||
SSTD_t *trans = Transaction_table; | |||
#else | |||
// recive transaction table index | |||
uint8_t tid; | |||
uint8_t pecount = 0; | |||
sync_recv(); | |||
tid = serial_read_chunk(&pecount,4); | |||
if(pecount> 0) | |||
return; | |||
serial_delay_half1(); | |||
serial_high(); // response step1 low->high | |||
serial_output(); | |||
_delay_sub_us(SLAVE_INT_ACK_WIDTH_UNIT*SLAVE_INT_ACK_WIDTH); | |||
SSTD_t *trans = &Transaction_table[tid]; | |||
serial_low(); // response step2 ack high->low | |||
#endif | |||
// target send phase | |||
if( trans->target2initiator_buffer_size > 0 ) | |||
serial_send_packet((uint8_t *)trans->target2initiator_buffer, | |||
trans->target2initiator_buffer_size); | |||
// target switch to input | |||
change_sender2reciver(); | |||
// target recive phase | |||
if( trans->initiator2target_buffer_size > 0 ) { | |||
if (serial_recive_packet((uint8_t *)trans->initiator2target_buffer, | |||
trans->initiator2target_buffer_size) ) { | |||
*trans->status = TRANSACTION_ACCEPTED; | |||
} else { | |||
*trans->status = TRANSACTION_DATA_ERROR; | |||
} | |||
} else { | |||
*trans->status = TRANSACTION_ACCEPTED; | |||
} | |||
sync_recv(); //weit initiator output to high | |||
} | |||
///////// | |||
// start transaction by initiator | |||
// | |||
// int soft_serial_transaction(int sstd_index) | |||
// | |||
// Returns: | |||
// TRANSACTION_END | |||
// TRANSACTION_NO_RESPONSE | |||
// TRANSACTION_DATA_ERROR | |||
// this code is very time dependent, so we need to disable interrupts | |||
#ifndef SERIAL_USE_MULTI_TRANSACTION | |||
int soft_serial_transaction(void) { | |||
SSTD_t *trans = Transaction_table; | |||
#else | |||
int soft_serial_transaction(int sstd_index) { | |||
SSTD_t *trans = &Transaction_table[sstd_index]; | |||
#endif | |||
cli(); | |||
// signal to the target that we want to start a transaction | |||
serial_output(); | |||
serial_low(); | |||
_delay_us(SLAVE_INT_WIDTH_US); | |||
#ifndef SERIAL_USE_MULTI_TRANSACTION | |||
// wait for the target response | |||
serial_input_with_pullup(); | |||
_delay_us(SLAVE_INT_RESPONSE_TIME); | |||
// check if the target is present | |||
if (serial_read_pin()) { | |||
// target failed to pull the line low, assume not present | |||
serial_output(); | |||
serial_high(); | |||
*trans->status = TRANSACTION_NO_RESPONSE; | |||
sei(); | |||
return TRANSACTION_NO_RESPONSE; | |||
} | |||
#else | |||
// send transaction table index | |||
sync_send(); | |||
_delay_sub_us(TID_SEND_ADJUST); | |||
serial_write_chunk(sstd_index, 4); | |||
serial_delay_half1(); | |||
// wait for the target response (step1 low->high) | |||
serial_input_with_pullup(); | |||
while( !serial_read_pin() ) { | |||
_delay_sub_us(2); | |||
} | |||
// check if the target is present (step2 high->low) | |||
for( int i = 0; serial_read_pin(); i++ ) { | |||
if (i > SLAVE_INT_ACK_WIDTH + 1) { | |||
// slave failed to pull the line low, assume not present | |||
serial_output(); | |||
serial_high(); | |||
*trans->status = TRANSACTION_NO_RESPONSE; | |||
sei(); | |||
return TRANSACTION_NO_RESPONSE; | |||
} | |||
_delay_sub_us(SLAVE_INT_ACK_WIDTH_UNIT); | |||
} | |||
#endif | |||
// initiator recive phase | |||
// if the target is present syncronize with it | |||
if( trans->target2initiator_buffer_size > 0 ) { | |||
if (!serial_recive_packet((uint8_t *)trans->target2initiator_buffer, | |||
trans->target2initiator_buffer_size) ) { | |||
serial_output(); | |||
serial_high(); | |||
*trans->status = TRANSACTION_DATA_ERROR; | |||
sei(); | |||
return TRANSACTION_DATA_ERROR; | |||
} | |||
} | |||
// initiator switch to output | |||
change_reciver2sender(); | |||
// initiator send phase | |||
if( trans->initiator2target_buffer_size > 0 ) { | |||
serial_send_packet((uint8_t *)trans->initiator2target_buffer, | |||
trans->initiator2target_buffer_size); | |||
} | |||
// always, release the line when not in use | |||
sync_send(); | |||
*trans->status = TRANSACTION_END; | |||
sei(); | |||
return TRANSACTION_END; | |||
} | |||
#ifdef SERIAL_USE_MULTI_TRANSACTION | |||
int soft_serial_get_and_clean_status(int sstd_index) { | |||
SSTD_t *trans = &Transaction_table[sstd_index]; | |||
cli(); | |||
int retval = *trans->status; | |||
*trans->status = 0;; | |||
sei(); | |||
return retval; | |||
} | |||
#endif | |||
#endif |
@ -0,0 +1,80 @@ | |||
#ifndef SOFT_SERIAL_H | |||
#define SOFT_SERIAL_H | |||
#include <stdbool.h> | |||
// ///////////////////////////////////////////////////////////////// | |||
// Need Soft Serial defines in serial_config.h | |||
// ///////////////////////////////////////////////////////////////// | |||
// ex. | |||
// #define SERIAL_PIN_DDR DDRD | |||
// #define SERIAL_PIN_PORT PORTD | |||
// #define SERIAL_PIN_INPUT PIND | |||
// #define SERIAL_PIN_MASK _BV(PD?) ?=0,2 | |||
// #define SERIAL_PIN_INTERRUPT INT?_vect ?=0,2 | |||
// | |||
// //// USE Simple API (OLD API, compatible with let's split serial.c) | |||
// ex. | |||
// #define SERIAL_SLAVE_BUFFER_LENGTH MATRIX_ROWS/2 | |||
// #define SERIAL_MASTER_BUFFER_LENGTH 1 | |||
// | |||
// //// USE flexible API (using multi-type transaction function) | |||
// #define SERIAL_USE_MULTI_TRANSACTION | |||
// | |||
// ///////////////////////////////////////////////////////////////// | |||
#ifndef SERIAL_USE_MULTI_TRANSACTION | |||
/* --- USE Simple API (OLD API, compatible with let's split serial.c) */ | |||
#if SERIAL_SLAVE_BUFFER_LENGTH > 0 | |||
extern volatile uint8_t serial_slave_buffer[SERIAL_SLAVE_BUFFER_LENGTH]; | |||
#endif | |||
#if SERIAL_MASTER_BUFFER_LENGTH > 0 | |||
extern volatile uint8_t serial_master_buffer[SERIAL_MASTER_BUFFER_LENGTH]; | |||
#endif | |||
void serial_master_init(void); | |||
void serial_slave_init(void); | |||
int serial_update_buffers(void); | |||
#endif // USE Simple API | |||
// Soft Serial Transaction Descriptor | |||
typedef struct _SSTD_t { | |||
uint8_t *status; | |||
uint8_t initiator2target_buffer_size; | |||
uint8_t *initiator2target_buffer; | |||
uint8_t target2initiator_buffer_size; | |||
uint8_t *target2initiator_buffer; | |||
} SSTD_t; | |||
// initiator is transaction start side | |||
void soft_serial_initiator_init(SSTD_t *sstd_table); | |||
// target is interrupt accept side | |||
void soft_serial_target_init(SSTD_t *sstd_table); | |||
// initiator resullt | |||
#define TRANSACTION_END 0 | |||
#define TRANSACTION_NO_RESPONSE 0x1 | |||
#define TRANSACTION_DATA_ERROR 0x2 | |||
#ifndef SERIAL_USE_MULTI_TRANSACTION | |||
int soft_serial_transaction(void); | |||
#else | |||
int soft_serial_transaction(int sstd_index); | |||
#endif | |||
// target status | |||
// *SSTD_t.status has | |||
// initiator: | |||
// TRANSACTION_END | |||
// or TRANSACTION_NO_RESPONSE | |||
// or TRANSACTION_DATA_ERROR | |||
// target: | |||
// TRANSACTION_DATA_ERROR | |||
// or TRANSACTION_ACCEPTED | |||
#define TRANSACTION_ACCEPTED 0x4 | |||
#ifdef SERIAL_USE_MULTI_TRANSACTION | |||
int soft_serial_get_and_clean_status(int sstd_index); | |||
#endif | |||
#endif /* SOFT_SERIAL_H */ |
@ -0,0 +1,8 @@ | |||
#define SERIAL_PIN_DDR DDRD | |||
#define SERIAL_PIN_PORT PORTD | |||
#define SERIAL_PIN_INPUT PIND | |||
#define SERIAL_PIN_MASK _BV(PD2) | |||
#define SERIAL_PIN_INTERRUPT INT2_vect | |||
#define SERIAL_SLAVE_BUFFER_LENGTH MATRIX_ROWS/2 | |||
#define SERIAL_MASTER_BUFFER_LENGTH 1 |
@ -0,0 +1,86 @@ | |||
#include <avr/io.h> | |||
#include <avr/wdt.h> | |||
#include <avr/power.h> | |||
#include <avr/interrupt.h> | |||
#include <util/delay.h> | |||
#include <avr/eeprom.h> | |||
#include "split_util.h" | |||
#include "matrix.h" | |||
#include "keyboard.h" | |||
#include "config.h" | |||
#include "timer.h" | |||
#ifdef USE_I2C | |||
# include "i2c.h" | |||
#else | |||
# include "serial.h" | |||
#endif | |||
volatile bool isLeftHand = true; | |||
static void setup_handedness(void) { | |||
#ifdef EE_HANDS | |||
isLeftHand = eeprom_read_byte(EECONFIG_HANDEDNESS); | |||
#else | |||
// I2C_MASTER_RIGHT is deprecated, use MASTER_RIGHT instead, since this works for both serial and i2c | |||
#if defined(I2C_MASTER_RIGHT) || defined(MASTER_RIGHT) | |||
isLeftHand = !has_usb(); | |||
#else | |||
isLeftHand = has_usb(); | |||
#endif | |||
#endif | |||
} | |||
static void keyboard_master_setup(void) { | |||
#ifdef USE_I2C | |||
i2c_master_init(); | |||
//#ifdef SSD1306OLED | |||
// matrix_master_OLED_init (); | |||
//#endif | |||
#else | |||
serial_master_init(); | |||
#endif | |||
} | |||
static void keyboard_slave_setup(void) { | |||
timer_init(); | |||
#ifdef USE_I2C | |||
i2c_slave_init(SLAVE_I2C_ADDRESS); | |||
#else | |||
serial_slave_init(); | |||
#endif | |||
} | |||
bool has_usb(void) { | |||
USBCON |= (1 << OTGPADE); //enables VBUS pad | |||
_delay_us(5); | |||
return (USBSTA & (1<<VBUS)); //checks state of VBUS | |||
} | |||
void split_keyboard_setup(void) { | |||
setup_handedness(); | |||
if (has_usb()) { | |||
keyboard_master_setup(); | |||
} else { | |||
keyboard_slave_setup(); | |||
} | |||
sei(); | |||
} | |||
void keyboard_slave_loop(void) { | |||
matrix_init(); | |||
while (1) { | |||
matrix_slave_scan(); | |||
} | |||
} | |||
// this code runs before the usb and keyboard is initialized | |||
void matrix_setup(void) { | |||
split_keyboard_setup(); | |||
if (!has_usb()) { | |||
keyboard_slave_loop(); | |||
} | |||
} |
@ -0,0 +1,20 @@ | |||
#ifndef SPLIT_KEYBOARD_UTIL_H | |||
#define SPLIT_KEYBOARD_UTIL_H | |||
#include <stdbool.h> | |||
#include "eeconfig.h" | |||
#define SLAVE_I2C_ADDRESS 0x32 | |||
extern volatile bool isLeftHand; | |||
// slave version of matix scan, defined in matrix.c | |||
void matrix_slave_scan(void); | |||
void split_keyboard_setup(void); | |||
bool has_usb(void); | |||
void keyboard_slave_loop(void); | |||
void matrix_master_OLED_init (void); | |||
#endif |
@ -0,0 +1,330 @@ | |||
#ifdef SSD1306OLED | |||
#include "ssd1306.h" | |||
#include "i2c.h" | |||
#include <string.h> | |||
#include "print.h" | |||
#include "glcdfont.c" | |||
#ifdef ADAFRUIT_BLE_ENABLE | |||
#include "adafruit_ble.h" | |||
#endif | |||
#ifdef PROTOCOL_LUFA | |||
#include "lufa.h" | |||
#endif | |||
#include "sendchar.h" | |||
#include "timer.h" | |||
// Set this to 1 to help diagnose early startup problems | |||
// when testing power-on with ble. Turn it off otherwise, | |||
// as the latency of printing most of the debug info messes | |||
// with the matrix scan, causing keys to drop. | |||
#define DEBUG_TO_SCREEN 0 | |||
//static uint16_t last_battery_update; | |||
//static uint32_t vbat; | |||
//#define BatteryUpdateInterval 10000 /* milliseconds */ | |||
#define ScreenOffInterval 300000 /* milliseconds */ | |||
#if DEBUG_TO_SCREEN | |||
static uint8_t displaying; | |||
#endif | |||
static uint16_t last_flush; | |||
// Write command sequence. | |||
// Returns true on success. | |||
static inline bool _send_cmd1(uint8_t cmd) { | |||
bool res = false; | |||
if (i2c_start_write(SSD1306_ADDRESS)) { | |||
xprintf("failed to start write to %d\n", SSD1306_ADDRESS); | |||
goto done; | |||
} | |||
if (i2c_master_write(0x0 /* command byte follows */)) { | |||
print("failed to write control byte\n"); | |||
goto done; | |||
} | |||
if (i2c_master_write(cmd)) { | |||
xprintf("failed to write command %d\n", cmd); | |||
goto done; | |||
} | |||
res = true; | |||
done: | |||
i2c_master_stop(); | |||
return res; | |||
} | |||
// Write 2-byte command sequence. | |||
// Returns true on success | |||
static inline bool _send_cmd2(uint8_t cmd, uint8_t opr) { | |||
if (!_send_cmd1(cmd)) { | |||
return false; | |||
} | |||
return _send_cmd1(opr); | |||
} | |||
// Write 3-byte command sequence. | |||
// Returns true on success | |||
static inline bool _send_cmd3(uint8_t cmd, uint8_t opr1, uint8_t opr2) { | |||
if (!_send_cmd1(cmd)) { | |||
return false; | |||
} | |||
if (!_send_cmd1(opr1)) { | |||
return false; | |||
} | |||
return _send_cmd1(opr2); | |||
} | |||
#define send_cmd1(c) if (!_send_cmd1(c)) {goto done;} | |||
#define send_cmd2(c,o) if (!_send_cmd2(c,o)) {goto done;} | |||
#define send_cmd3(c,o1,o2) if (!_send_cmd3(c,o1,o2)) {goto done;} | |||
static void clear_display(void) { | |||
matrix_clear(&display); | |||
// Clear all of the display bits (there can be random noise | |||
// in the RAM on startup) | |||
send_cmd3(PageAddr, 0, (DisplayHeight / 8) - 1); | |||
send_cmd3(ColumnAddr, 0, DisplayWidth - 1); | |||
if (i2c_start_write(SSD1306_ADDRESS)) { | |||
goto done; | |||
} | |||
if (i2c_master_write(0x40)) { | |||
// Data mode | |||
goto done; | |||
} | |||
for (uint8_t row = 0; row < MatrixRows; ++row) { | |||
for (uint8_t col = 0; col < DisplayWidth; ++col) { | |||
i2c_master_write(0); | |||
} | |||
} | |||
display.dirty = false; | |||
done: | |||
i2c_master_stop(); | |||
} | |||
#if DEBUG_TO_SCREEN | |||
#undef sendchar | |||
static int8_t capture_sendchar(uint8_t c) { | |||
sendchar(c); | |||
iota_gfx_write_char(c); | |||
if (!displaying) { | |||
iota_gfx_flush(); | |||
} | |||
return 0; | |||
} | |||
#endif | |||
bool iota_gfx_init(bool rotate) { | |||
bool success = false; | |||
send_cmd1(DisplayOff); | |||
send_cmd2(SetDisplayClockDiv, 0x80); | |||
send_cmd2(SetMultiPlex, DisplayHeight - 1); | |||
send_cmd2(SetDisplayOffset, 0); | |||
send_cmd1(SetStartLine | 0x0); | |||
send_cmd2(SetChargePump, 0x14 /* Enable */); | |||
send_cmd2(SetMemoryMode, 0 /* horizontal addressing */); | |||
if(rotate){ | |||
// the following Flip the display orientation 180 degrees | |||
send_cmd1(SegRemap); | |||
send_cmd1(ComScanInc); | |||
}else{ | |||
// Flips the display orientation 0 degrees | |||
send_cmd1(SegRemap | 0x1); | |||
send_cmd1(ComScanDec); | |||
} | |||
send_cmd2(SetComPins, 0x2); | |||
send_cmd2(SetContrast, 0x8f); | |||
send_cmd2(SetPreCharge, 0xf1); | |||
send_cmd2(SetVComDetect, 0x40); | |||
send_cmd1(DisplayAllOnResume); | |||
send_cmd1(NormalDisplay); | |||
send_cmd1(DeActivateScroll); | |||
send_cmd1(DisplayOn); | |||
send_cmd2(SetContrast, 0); // Dim | |||
clear_display(); | |||
success = true; | |||
iota_gfx_flush(); | |||
#if DEBUG_TO_SCREEN | |||
print_set_sendchar(capture_sendchar); | |||
#endif | |||
done: | |||
return success; | |||
} | |||
bool iota_gfx_off(void) { | |||
bool success = false; | |||
send_cmd1(DisplayOff); | |||
success = true; | |||
done: | |||
return success; | |||
} | |||
bool iota_gfx_on(void) { | |||
bool success = false; | |||
send_cmd1(DisplayOn); | |||
success = true; | |||
done: | |||
return success; | |||
} | |||
void matrix_write_char_inner(struct CharacterMatrix *matrix, uint8_t c) { | |||
*matrix->cursor = c; | |||
++matrix->cursor; | |||
if (matrix->cursor - &matrix->display[0][0] == sizeof(matrix->display)) { | |||
// We went off the end; scroll the display upwards by one line | |||
memmove(&matrix->display[0], &matrix->display[1], | |||
MatrixCols * (MatrixRows - 1)); | |||
matrix->cursor = &matrix->display[MatrixRows - 1][0]; | |||
memset(matrix->cursor, ' ', MatrixCols); | |||
} | |||
} | |||
void matrix_write_char(struct CharacterMatrix *matrix, uint8_t c) { | |||
matrix->dirty = true; | |||
if (c == '\n') { | |||
// Clear to end of line from the cursor and then move to the | |||
// start of the next line | |||
uint8_t cursor_col = (matrix->cursor - &matrix->display[0][0]) % MatrixCols; | |||
while (cursor_col++ < MatrixCols) { | |||
matrix_write_char_inner(matrix, ' '); | |||
} | |||
return; | |||
} | |||
matrix_write_char_inner(matrix, c); | |||
} | |||
void iota_gfx_write_char(uint8_t c) { | |||
matrix_write_char(&display, c); | |||
} | |||
void matrix_write(struct CharacterMatrix *matrix, const char *data) { | |||
const char *end = data + strlen(data); | |||
while (data < end) { | |||
matrix_write_char(matrix, *data); | |||
++data; | |||
} | |||
} | |||
void matrix_write_ln(struct CharacterMatrix *matrix, const char *data) { | |||
char data_ln[strlen(data)+2]; | |||
snprintf(data_ln, sizeof(data_ln), "%s\n", data); | |||
matrix_write(matrix, data_ln); | |||
} | |||
void iota_gfx_write(const char *data) { | |||
matrix_write(&display, data); | |||
} | |||
void matrix_write_P(struct CharacterMatrix *matrix, const char *data) { | |||
while (true) { | |||
uint8_t c = pgm_read_byte(data); | |||
if (c == 0) { | |||
return; | |||
} | |||
matrix_write_char(matrix, c); | |||
++data; | |||
} | |||
} | |||
void iota_gfx_write_P(const char *data) { | |||
matrix_write_P(&display, data); | |||
} | |||
void matrix_clear(struct CharacterMatrix *matrix) { | |||
memset(matrix->display, ' ', sizeof(matrix->display)); | |||
matrix->cursor = &matrix->display[0][0]; | |||
matrix->dirty = true; | |||
} | |||
void iota_gfx_clear_screen(void) { | |||
matrix_clear(&display); | |||
} | |||
void matrix_render(struct CharacterMatrix *matrix) { | |||
last_flush = timer_read(); | |||
iota_gfx_on(); | |||
#if DEBUG_TO_SCREEN | |||
++displaying; | |||
#endif | |||
// Move to the home position | |||
send_cmd3(PageAddr, 0, MatrixRows - 1); | |||
send_cmd3(ColumnAddr, 0, (MatrixCols * FontWidth) - 1); | |||
if (i2c_start_write(SSD1306_ADDRESS)) { | |||
goto done; | |||
} | |||
if (i2c_master_write(0x40)) { | |||
// Data mode | |||
goto done; | |||
} | |||
for (uint8_t row = 0; row < MatrixRows; ++row) { | |||
for (uint8_t col = 0; col < MatrixCols; ++col) { | |||
const uint8_t *glyph = font + (matrix->display[row][col] * FontWidth); | |||
for (uint8_t glyphCol = 0; glyphCol < FontWidth; ++glyphCol) { | |||
uint8_t colBits = pgm_read_byte(glyph + glyphCol); | |||
i2c_master_write(colBits); | |||
} | |||
// 1 column of space between chars (it's not included in the glyph) | |||
//i2c_master_write(0); | |||
} | |||
} | |||
matrix->dirty = false; | |||
done: | |||
i2c_master_stop(); | |||
#if DEBUG_TO_SCREEN | |||
--displaying; | |||
#endif | |||
} | |||
void iota_gfx_flush(void) { | |||
matrix_render(&display); | |||
} | |||
__attribute__ ((weak)) | |||
void iota_gfx_task_user(void) { | |||
} | |||
void iota_gfx_task(void) { | |||
iota_gfx_task_user(); | |||
if (display.dirty) { | |||
iota_gfx_flush(); | |||
} | |||
if (timer_elapsed(last_flush) > ScreenOffInterval) { | |||
iota_gfx_off(); | |||
} | |||
} | |||
#endif |
@ -0,0 +1,94 @@ | |||
#ifndef SSD1306_H | |||
#define SSD1306_H | |||
#include <stdbool.h> | |||
#include <stdio.h> | |||
#include "pincontrol.h" | |||
#include "config.h" | |||
enum ssd1306_cmds { | |||
DisplayOff = 0xAE, | |||
DisplayOn = 0xAF, | |||
SetContrast = 0x81, | |||
DisplayAllOnResume = 0xA4, | |||
DisplayAllOn = 0xA5, | |||
NormalDisplay = 0xA6, | |||
InvertDisplay = 0xA7, | |||
SetDisplayOffset = 0xD3, | |||
SetComPins = 0xda, | |||
SetVComDetect = 0xdb, | |||
SetDisplayClockDiv = 0xD5, | |||
SetPreCharge = 0xd9, | |||
SetMultiPlex = 0xa8, | |||
SetLowColumn = 0x00, | |||
SetHighColumn = 0x10, | |||
SetStartLine = 0x40, | |||
SetMemoryMode = 0x20, | |||
ColumnAddr = 0x21, | |||
PageAddr = 0x22, | |||
ComScanInc = 0xc0, | |||
ComScanDec = 0xc8, | |||
SegRemap = 0xa0, | |||
SetChargePump = 0x8d, | |||
ExternalVcc = 0x01, | |||
SwitchCapVcc = 0x02, | |||
ActivateScroll = 0x2f, | |||
DeActivateScroll = 0x2e, | |||
SetVerticalScrollArea = 0xa3, | |||
RightHorizontalScroll = 0x26, | |||
LeftHorizontalScroll = 0x27, | |||
VerticalAndRightHorizontalScroll = 0x29, | |||
VerticalAndLeftHorizontalScroll = 0x2a, | |||
}; | |||
// Controls the SSD1306 128x32 OLED display via i2c | |||
#ifndef SSD1306_ADDRESS | |||
#define SSD1306_ADDRESS 0x3C | |||
#endif | |||
#define DisplayHeight 32 | |||
#define DisplayWidth 128 | |||
#define FontHeight 8 | |||
#define FontWidth 6 | |||
#define MatrixRows (DisplayHeight / FontHeight) | |||
#define MatrixCols (DisplayWidth / FontWidth) | |||
struct CharacterMatrix { | |||
uint8_t display[MatrixRows][MatrixCols]; | |||
uint8_t *cursor; | |||
bool dirty; | |||
}; | |||
struct CharacterMatrix display; | |||
bool iota_gfx_init(bool rotate); | |||
void iota_gfx_task(void); | |||
bool iota_gfx_off(void); | |||
bool iota_gfx_on(void); | |||
void iota_gfx_flush(void); | |||
void iota_gfx_write_char(uint8_t c); | |||
void iota_gfx_write(const char *data); | |||
void iota_gfx_write_P(const char *data); | |||
void iota_gfx_clear_screen(void); | |||
void iota_gfx_task_user(void); | |||
void matrix_clear(struct CharacterMatrix *matrix); | |||
void matrix_write_char_inner(struct CharacterMatrix *matrix, uint8_t c); | |||
void matrix_write_char(struct CharacterMatrix *matrix, uint8_t c); | |||
void matrix_write(struct CharacterMatrix *matrix, const char *data); | |||
void matrix_write_ln(struct CharacterMatrix *matrix, const char *data); | |||
void matrix_write_P(struct CharacterMatrix *matrix, const char *data); | |||
void matrix_render(struct CharacterMatrix *matrix); | |||
#endif |