diff --git a/keyboards/halfcliff/config.h b/keyboards/halfcliff/config.h
new file mode 100644
index 00000000000..287fd2f4ac7
--- /dev/null
+++ b/keyboards/halfcliff/config.h
@@ -0,0 +1,69 @@
+/*
+Copyright 2021 n2
+
+This program is free software: you can redistribute it and/or modify
+it under the terms of the GNU General Public License as published by
+the Free Software Foundation, either version 2 of the License, or
+(at your option) any later version.
+
+This program is distributed in the hope that it will be useful,
+but WITHOUT ANY WARRANTY; without even the implied warranty of
+MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
+GNU General Public License for more details.
+
+You should have received a copy of the GNU General Public License
+along with this program. If not, see .
+*/
+
+#pragma once
+
+#include "config_common.h"
+
+/* USB Device descriptor parameter */
+#define VENDOR_ID 0x0335
+#define PRODUCT_ID 0x0021
+#define DEVICE_VER 0x0001
+#define MANUFACTURER n2
+#define PRODUCT halfcliff
+
+/* key matrix size */
+#define MATRIX_ROWS 20
+#define MATRIX_COLS 5
+
+#define MATRIX_ROW_PINS { F5, F6, F7, D7, B5, F5, F6, F7, D7, B5 }
+#define MATRIX_COL_PINS { B4, E6, C6, B6, B2 }
+#define UNUSED_PINS
+
+//#define NUMBER_OF_ENCODERS 1
+#define ENCODERS_PAD_A { D4 }
+#define ENCODERS_PAD_B { F4 }
+#define ENCODER_RESOLUTION 2
+
+/*
+ * Split Keyboard specific options, make sure you have 'SPLIT_KEYBOARD = yes' in your rules.mk, and define SOFT_SERIAL_PIN.
+ */
+#define SOFT_SERIAL_PIN D2 // or D1, D2, D3, E6
+
+ #define RGB_DI_PIN D3
+ #ifdef RGB_DI_PIN
+ #define RGBLED_NUM 10
+ #define RGBLIGHT_SPLIT
+ #define RGBLED_SPLIT { 5, 5 }
+ #define RGBLIGHT_HUE_STEP 8
+ #define RGBLIGHT_SAT_STEP 8
+ #define RGBLIGHT_VAL_STEP 8
+ #define RGBLIGHT_LIMIT_VAL 255 /* The maximum brightness level */
+ #define RGBLIGHT_SLEEP /* If defined, the RGB lighting will be switched off when the host goes to sleep */
+ #endif
+
+/* Debounce reduces chatter (unintended double-presses) - set 0 if debouncing is not needed */
+#define DEBOUNCE 5
+
+/* Mechanical locking support. Use KC_LCAP, KC_LNUM or KC_LSCR instead in keymap */
+#define LOCKING_SUPPORT_ENABLE
+/* Locking resynchronize hack */
+#define LOCKING_RESYNC_ENABLE
+
+/* disable these deprecated features by default */
+#define NO_ACTION_MACRO
+#define NO_ACTION_FUNCTION
diff --git a/keyboards/halfcliff/halfcliff.c b/keyboards/halfcliff/halfcliff.c
new file mode 100644
index 00000000000..999e9036db5
--- /dev/null
+++ b/keyboards/halfcliff/halfcliff.c
@@ -0,0 +1,92 @@
+/* Copyright 2021 n2
+ *
+ * This program is free software: you can redistribute it and/or modify
+ * it under the terms of the GNU General Public License as published by
+ * the Free Software Foundation, either version 2 of the License, or
+ * (at your option) any later version.
+ *
+ * This program is distributed in the hope that it will be useful,
+ * but WITHOUT ANY WARRANTY; without even the implied warranty of
+ * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
+ * GNU General Public License for more details.
+ *
+ * You should have received a copy of the GNU General Public License
+ * along with this program. If not, see .
+ */
+
+#include "halfcliff.h"
+
+#ifdef OLED_DRIVER_ENABLE
+
+// Defines names for use in layer keycodes and the keymap
+enum layer_names {
+ _DEFAULT = 0,
+ _RAISE,
+ _LOWER,
+ _ADJUST
+/* _FN */
+};
+
+__attribute__((weak)) oled_rotation_t oled_init_user(oled_rotation_t rotation) {
+ if (!is_keyboard_master()) {
+ return OLED_ROTATION_180; // flips the display 180 degrees if offhand
+ }
+ return rotation;
+}
+
+__attribute__((weak)) void oled_task_user(void) {
+ if (!is_keyboard_master()) {
+ static const char PROGMEM qmk_logo[] = {
+ 0x80, 0x81, 0x82, 0x83, 0x84, 0x85, 0x86, 0x87, 0x88, 0x89, 0x8A, 0x8B, 0x8C, 0x8D, 0x8E, 0x8F, 0x90, 0x91, 0x92, 0x93, 0x94,
+ 0xA0, 0xA1, 0xA2, 0xA3, 0xA4, 0xA5, 0xA6, 0xA7, 0xA8, 0xA9, 0xAA, 0xAB, 0xAC, 0xAD, 0xAE, 0xAF, 0xB0, 0xB1, 0xB2, 0xB3, 0xB4,
+ 0xC0, 0xC1, 0xC2, 0xC3, 0xC4, 0xC5, 0xC6, 0xC7, 0xC8, 0xC9, 0xCA, 0xCB, 0xCC, 0xCD, 0xCE, 0xCF, 0xD0, 0xD1, 0xD2, 0xD3, 0xD4, 0x00
+ };
+ oled_write_P(qmk_logo, false);
+ } else {
+ // Host Keyboard Layer Status
+ oled_write_P(PSTR("Layer: "), false);
+ switch (get_highest_layer(layer_state)) {
+ case _DEFAULT:
+ oled_write_P(PSTR("DEFAULT\n"), false);
+ break;
+ case _RAISE:
+ oled_write_P(PSTR("RAISE\n"), false);
+ break;
+ case _LOWER:
+ oled_write_P(PSTR("LOWER\n"), false);
+ break;
+ case _ADJUST:
+ oled_write_P(PSTR("ADJUST\n"), false);
+ break;
+ default:
+ // Or use the write_ln shortcut over adding '\n' to the end of your string
+ oled_write_ln_P(PSTR("Undefined"), false);
+ };
+ // Host Keyboard LED Status
+ led_t led_state = host_keyboard_led_state();
+ oled_write_P(led_state.num_lock ? PSTR("NUMLCK ") : PSTR(" "), false);
+ oled_write_P(led_state.caps_lock ? PSTR("CAPLCK ") : PSTR(" "), false);
+ oled_write_P(led_state.scroll_lock ? PSTR("SCRLCK\n") : PSTR(" \n"), false);
+ }
+}
+#endif
+
+#ifdef ENCODER_ENABLE
+bool encoder_update_kb(uint8_t index, bool clockwise) {
+ if (!encoder_update_user(index, clockwise)) { return false; }
+ if (index == 0) { /* Left side encoder */
+ if (clockwise) {
+ tap_code(KC_WH_U);
+ } else {
+ tap_code(KC_WH_D);
+ }
+ } else if (index == 1) { /* Right side encoder */
+ if (clockwise) {
+ tap_code(KC_WH_U);
+ } else {
+ tap_code(KC_WH_D);
+ }
+ }
+ return true;
+}
+#endif
diff --git a/keyboards/halfcliff/halfcliff.h b/keyboards/halfcliff/halfcliff.h
new file mode 100644
index 00000000000..6e4c808641e
--- /dev/null
+++ b/keyboards/halfcliff/halfcliff.h
@@ -0,0 +1,58 @@
+/* Copyright 2021 n2
+ *
+ * This program is free software: you can redistribute it and/or modify
+ * it under the terms of the GNU General Public License as published by
+ * the Free Software Foundation, either version 2 of the License, or
+ * (at your option) any later version.
+ *
+ * This program is distributed in the hope that it will be useful,
+ * but WITHOUT ANY WARRANTY; without even the implied warranty of
+ * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
+ * GNU General Public License for more details.
+ *
+ * You should have received a copy of the GNU General Public License
+ * along with this program. If not, see .
+ */
+
+#pragma once
+
+#include "quantum.h"
+
+/* This is a shortcut to help you visually see your layout.
+ *
+ * The first section contains all of the arguments representing the physical
+ * layout of the board and position of the keys.
+ *
+ * The second converts the arguments into a two-dimensional array which
+ * represents the switch matrix.
+ */
+
+#define LAYOUT( \
+ l51,l50,l00,l01,l02,l03,l04,r04,r03,r02,r01,r00,r50,r51,r52,\
+ l61,l60,l10,l11,l12,l13,l14,r14,r13,r12,r11,r10,r60,r61,r62,\
+ l71,l70,l20,l21,l22,l23,l24,r24,r23,r22,r21,r20,r70,r71,r72,\
+ l81,l80,l30,l31,l32,l33,l34,r34,r33,r32,r31,r30,r80,r81,r82,\
+ l91,l90,l40,l41,l42,l43,l44,r44,r43,r42,r41,r40,r90,r91,r92\
+) \
+{ \
+ {l00, l01, l02, l03, l04 }, \
+ {l10, l11, l12, l13, l14 }, \
+ {l20, l21, l22, l23, l24 }, \
+ {l30, l31, l32, l33, l34 }, \
+ {l40, l41, l42, l43, l44 }, \
+ {l50, l51, KC_NO,KC_NO,KC_NO}, \
+ {l60, l61, KC_NO,KC_NO,KC_NO}, \
+ {l70, l71, KC_NO,KC_NO,KC_NO}, \
+ {l80, l81, KC_NO,KC_NO,KC_NO}, \
+ {l90, l91, KC_NO,KC_NO,KC_NO}, \
+ {r00, r01, r02, r03, r04 }, \
+ {r10, r11, r12, r13, r14 }, \
+ {r20, r21, r22, r23, r24 }, \
+ {r30, r31, r32, r33, r34 }, \
+ {r40, r41, r42, r43, r44 }, \
+ {r50, r51, r52, KC_NO,KC_NO}, \
+ {r60, r61, r62, KC_NO,KC_NO}, \
+ {r70, r71, r72, KC_NO,KC_NO}, \
+ {r80, r81, r82, KC_NO,KC_NO}, \
+ {r90, r91, r92, KC_NO,KC_NO}, \
+}
diff --git a/keyboards/halfcliff/info.json b/keyboards/halfcliff/info.json
new file mode 100644
index 00000000000..2a859bb4c3c
--- /dev/null
+++ b/keyboards/halfcliff/info.json
@@ -0,0 +1,88 @@
+{
+ "keyboard_name": "halfcliff",
+ "url": "https://github.com/N2-Sumikko",
+ "maintainer": "n2",
+ "width": 17.25,
+ "height": 5,
+ "layouts": {
+ "LAYOUT": {
+ "layout": [
+ {"x":0.75,"y":0},
+ {"x":1.75,"y":0},
+ {"x":2.75,"y":0},
+ {"x":3.75,"y":0},
+ {"x":4.75,"y":0},
+ {"x":5.75,"y":0},
+ {"x":6.75,"y":0},
+ {"x":8.25,"y":0},
+ {"x":9.25,"y":0},
+ {"x":10.25,"y":0},
+ {"x":11.25,"y":0},
+ {"x":12.25,"y":0},
+ {"x":13.25,"y":0},
+ {"x":14.25,"y":0},
+ {"x":15.25,"y":0},
+ {"x":0.25,"y":1,"w":1.5},
+ {"x":1.75,"y":1},
+ {"x":2.75,"y":1},
+ {"x":3.75,"y":1},
+ {"x":4.75,"y":1},
+ {"x":5.75,"y":1},
+ {"x":6.75,"y":1},
+ {"x":8.25,"y":1},
+ {"x":9.25,"y":1},
+ {"x":10.25,"y":1},
+ {"x":11.25,"y":1},
+ {"x":12.25,"y":1},
+ {"x":13.25,"y":1},
+ {"x":14.25,"y":1},
+ {"x":16.25,"y":0},
+ {"x":0,"y":2,"w":1.75},
+ {"x":1.75,"y":2},
+ {"x":2.75,"y":2},
+ {"x":3.75,"y":2},
+ {"x":4.75,"y":2},
+ {"x":5.75,"y":2},
+ {"x":6.75,"y":2},
+ {"x":8.25,"y":2},
+ {"x":9.25,"y":2},
+ {"x":10.25,"y":2},
+ {"x":11.25,"y":2},
+ {"x":12.25,"y":2},
+ {"x":13.25,"y":2},
+ {"x":14.25,"y":2},
+ {"x":15.5,"y":1,"w":1.25,"h":2},
+ {"x":0,"y":3,"w":1.75},
+ {"x":1.75,"y":3},
+ {"x":2.75,"y":3},
+ {"x":3.75,"y":3},
+ {"x":4.75,"y":3},
+ {"x":5.75,"y":3},
+ {"x":6.75,"y":3},
+ {"x":8.25,"y":3},
+ {"x":9.25,"y":3},
+ {"x":10.25,"y":3},
+ {"x":11.25,"y":3},
+ {"x":12.25,"y":3},
+ {"x":13.25,"y":3},
+ {"x":14.25,"y":3},
+ {"x":15.25,"y":3,"w":2},
+ {"x":0.5,"y":4,"w":1.25},
+ {"x":1.75,"y":4},
+ {"x":2.75,"y":4},
+ {"x":3.75,"y":4},
+ {"x":4.75,"y":4},
+ {"x":5.75,"y":4},
+ {"x":6.75,"y":4},
+ {"x":8.25,"y":4},
+ {"x":9.25,"y":4},
+ {"x":10.25,"y":4},
+ {"x":11.25,"y":4},
+ {"x":12.25,"y":4},
+ {"x":13.25,"y":4},
+ {"x":14.25,"y":4},
+ {"x":15.25,"y":4}
+ ]
+ }
+ }
+}
\ No newline at end of file
diff --git a/keyboards/halfcliff/keymaps/default/keymap.c b/keyboards/halfcliff/keymaps/default/keymap.c
new file mode 100644
index 00000000000..02743f5d494
--- /dev/null
+++ b/keyboards/halfcliff/keymaps/default/keymap.c
@@ -0,0 +1,109 @@
+/* Copyright 2021 n2
+ *
+ * This program is free software: you can redistribute it and/or modify
+ * it under the terms of the GNU General Public License as published by
+ * the Free Software Foundation, either version 2 of the License, or
+ * (at your option) any later version.
+ *
+ * This program is distributed in the hope that it will be useful,
+ * but WITHOUT ANY WARRANTY; without even the implied warranty of
+ * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
+ * GNU General Public License for more details.
+ *
+ * You should have received a copy of the GNU General Public License
+ * along with this program. If not, see .
+ */
+#include QMK_KEYBOARD_H
+#include "keymap_jp.h"
+
+// Defines names for use in layer keycodes and the keymap
+enum layer_names {
+ _DEFAULT = 0,
+ _RAISE,
+ _LOWER,
+ _ADJUST
+/* _FN */
+};
+
+const uint16_t PROGMEM keymaps[][MATRIX_ROWS][MATRIX_COLS] = {
+ /* DEFAULT
+ * ,--------------------------------------------------------------------------------------------------------------------------------------.
+ * |ZEN/HAN | 1 | 2 | 3 | 4 | 5 | | 6 | 7 | 8 | 9 | 0 | - | ^ | \ |
+ * |--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------|
+ * | TAB | q | w | e | r | t | | y | u | i | o | p | @ | [ | BSPC |
+ * |--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------|
+ * | CAPS | a | s | d | f | g | | h | j | k | l | ; | : | ] | ENT |
+ * |--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------|
+ * | LSHIFT | z | x | c | v | b | | n | m | , | . | / | \ | UP | RSHIFT |
+ * |--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------|
+ * | CTRL | LGUI | ALT | MUHEN | SPACE | SPACE | LOWER | RAISE | SPACE | HENKAN | KANA | APP | LEFT | DOWN | RIGHT |
+ * `--------------------------------------------------------------------------------------------------------------------------------------'
+ */
+ [_DEFAULT] = LAYOUT(
+ KC_ZKHK, KC_1, KC_2, KC_3, KC_4, KC_5, _______, KC_6, KC_7, KC_8, KC_9, KC_0, JP_MINS, JP_CIRC, JP_YEN,
+ KC_TAB, KC_Q, KC_W, KC_E, KC_R, KC_T, _______, KC_Y, KC_U, KC_I, KC_O, KC_P, JP_AT , JP_LBRC, KC_BSPC,
+ KC_CAPS, KC_A, KC_S, KC_D, KC_F, KC_G, _______, KC_H, KC_J, KC_K, KC_L, KC_SCLN, JP_COLN, JP_RBRC, KC_ENT,
+ KC_LSFT, KC_Z, KC_X, KC_C, KC_V, KC_B, _______, KC_N, KC_M, KC_COMM, KC_DOT, KC_SLSH, JP_BSLS, KC_UP, KC_RSFT,
+ KC_LCTL, KC_LGUI, KC_LALT, KC_MHEN, KC_SPC, KC_SPC, MO(_LOWER), MO(_RAISE), KC_SPC, KC_HENK, KC_KANA, KC_APP, KC_LEFT, KC_DOWN, KC_RGHT
+ ),
+ /* RAISE
+ * ,--------------------------------------------------------------------------------------------------------------------------------------.
+ * | | | | | | | | | | | | | | | |
+ * |--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------|
+ * | | 1 | 2 | 3 | 4 | 5 | | 6 | 7 | 8 | 9 | 0 | | | |
+ * |--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------|
+ * | | | | | | | | | | | | | | | |
+ * |--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------|
+ * | | | | | | | | | | | | | | | |
+ * |--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------|
+ * | | | | | | | | | | | | | | | |
+ * `--------------------------------------------------------------------------------------------------------------------------------------'
+ */
+ [_RAISE] = LAYOUT(
+ _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
+ _______, KC_1, KC_2, KC_3, KC_4, KC_5, _______, KC_6, KC_7, KC_8, KC_9, KC_0, _______, _______, _______,
+ _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
+ _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
+ _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______
+ ),
+ /* LOWER
+ * ,--------------------------------------------------------------------------------------------------------------------------------------.
+ * | | | | | | |RGB_TOG | | | | | | | | |
+ * |--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------|
+ * | | | | | | |RGB_VAI | | | | | | | | |
+ * |--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------|
+ * | | | | | | |RGB_HUI | | | | | | | | |
+ * |--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------|
+ * | | | | | | |RGB_SAI | | | | | | | | |
+ * |--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------|
+ * | | | | | | | | | | | | | | | |
+ * `--------------------------------------------------------------------------------------------------------------------------------------'
+ */
+ [_LOWER] = LAYOUT(
+ _______, _______, _______, _______, _______, _______, RGB_TOG, _______, _______, _______, _______, _______, _______, _______, _______,
+ _______, _______, _______, _______, _______, _______, RGB_VAI, _______, _______, _______, _______, _______, _______, _______, _______,
+ _______, _______, _______, _______, _______, _______, RGB_HUI, _______, _______, _______, _______, _______, _______, _______, _______,
+ _______, _______, _______, _______, _______, _______, RGB_SAI, _______, _______, _______, _______, _______, _______, _______, _______,
+ _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______
+ ),
+ /* ADJUST
+ * ,--------------------------------------------------------------------------------------------------------------------------------------.
+ * | | | | | | | | | | | | | | | |
+ * |--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------|
+ * | | | | | | | | | | | | | | | |
+ * |--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------|
+ * | | | | | | | | | | | | | | | |
+ * |--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------|
+ * | | | | | | | | | | | | | | | |
+ * |--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------|
+ * | | | | | | | | | | | | | | | |
+ * `--------------------------------------------------------------------------------------------------------------------------------------'
+ */
+ [_ADJUST] = LAYOUT(
+ _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
+ _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
+ _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
+ _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
+ _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______
+ )
+};
diff --git a/keyboards/halfcliff/keymaps/default/readme.md b/keyboards/halfcliff/keymaps/default/readme.md
new file mode 100644
index 00000000000..2924c68c24a
--- /dev/null
+++ b/keyboards/halfcliff/keymaps/default/readme.md
@@ -0,0 +1 @@
+# The default keymap for halfcliff
diff --git a/keyboards/halfcliff/keymaps/via/keymap.c b/keyboards/halfcliff/keymaps/via/keymap.c
new file mode 100644
index 00000000000..e0a825e7c30
--- /dev/null
+++ b/keyboards/halfcliff/keymaps/via/keymap.c
@@ -0,0 +1,112 @@
+/* Copyright 2021 n2
+ *
+ * This program is free software: you can redistribute it and/or modify
+ * it under the terms of the GNU General Public License as published by
+ * the Free Software Foundation, either version 2 of the License, or
+ * (at your option) any later version.
+ *
+ * This program is distributed in the hope that it will be useful,
+ * but WITHOUT ANY WARRANTY; without even the implied warranty of
+ * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
+ * GNU General Public License for more details.
+ *
+ * You should have received a copy of the GNU General Public License
+ * along with this program. If not, see .
+ */
+#include QMK_KEYBOARD_H
+#include "keymap_jp.h"
+
+#define RAISE FN_MO13
+#define LOWER FN_MO23
+
+// Defines names for use in layer keycodes and the keymap
+enum layer_names {
+ _DEFAULT = 0,
+ _RAISE,
+ _LOWER,
+ _ADJUST
+/* _FN */
+};
+
+const uint16_t PROGMEM keymaps[][MATRIX_ROWS][MATRIX_COLS] = {
+ /* DEFAULT
+ * ,--------------------------------------------------------------------------------------------------------------------------------------.
+ * |ZEN/HAN | 1 | 2 | 3 | 4 | 5 | | 6 | 7 | 8 | 9 | 0 | - | ^ | \ |
+ * |--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------|
+ * | TAB | q | w | e | r | t | | y | u | i | o | p | @ | [ | BSPC |
+ * |--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------|
+ * | CAPS | a | s | d | f | g | | h | j | k | l | ; | : | ] | ENT |
+ * |--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------|
+ * | LSHIFT | z | x | c | v | b | | n | m | , | . | / | \ | UP | RSHIFT |
+ * |--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------|
+ * | CTRL | LGUI | ALT | MUHEN | SPACE | SPACE | LOWER | RAISE | SPACE | HENKAN | KANA | APP | LEFT | DOWN | RIGHT |
+ * `--------------------------------------------------------------------------------------------------------------------------------------'
+ */
+ [_DEFAULT] = LAYOUT(
+ KC_ZKHK, KC_1, KC_2, KC_3, KC_4, KC_5, _______, KC_6, KC_7, KC_8, KC_9, KC_0, JP_MINS, JP_CIRC, JP_YEN,
+ KC_TAB, KC_Q, KC_W, KC_E, KC_R, KC_T, _______, KC_Y, KC_U, KC_I, KC_O, KC_P, JP_AT , JP_LBRC, KC_BSPC,
+ KC_CAPS, KC_A, KC_S, KC_D, KC_F, KC_G, _______, KC_H, KC_J, KC_K, KC_L, KC_SCLN, JP_COLN, JP_RBRC, KC_ENT,
+ KC_LSFT, KC_Z, KC_X, KC_C, KC_V, KC_B, _______, KC_N, KC_M, KC_COMM, KC_DOT, KC_SLSH, JP_BSLS, KC_UP, KC_RSFT,
+ KC_LCTL, KC_LGUI, KC_LALT, KC_MHEN, KC_SPC, KC_SPC, FN_MO23, FN_MO13, KC_SPC, KC_HENK, KC_KANA, KC_APP, KC_LEFT, KC_DOWN, KC_RGHT
+ ),
+ /* RAISE
+ * ,--------------------------------------------------------------------------------------------------------------------------------------.
+ * | | | | | | | | | | | | | | | |
+ * |--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------|
+ * | | 1 | 2 | 3 | 4 | 5 | | 6 | 7 | 8 | 9 | 0 | | | |
+ * |--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------|
+ * | | | | | | | | | | | | | | | |
+ * |--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------|
+ * | | | | | | | | | | | | | | | |
+ * |--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------|
+ * | | | | | | | | | | | | | | | |
+ * `--------------------------------------------------------------------------------------------------------------------------------------'
+ */
+ [_RAISE] = LAYOUT(
+ _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
+ _______, KC_1, KC_2, KC_3, KC_4, KC_5, _______, KC_6, KC_7, KC_8, KC_9, KC_0, _______, _______, _______,
+ _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
+ _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
+ _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______
+ ),
+ /* LOWER
+ * ,--------------------------------------------------------------------------------------------------------------------------------------.
+ * | | | | | | |RGB_TOG | | | | | | | | |
+ * |--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------|
+ * | | | | | | |RGB_VAI | | | | | | | | |
+ * |--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------|
+ * | | | | | | |RGB_HUI | | | | | | | | |
+ * |--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------|
+ * | | | | | | |RGB_SAI | | | | | | | | |
+ * |--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------|
+ * | | | | | | | | | | | | | | | |
+ * `--------------------------------------------------------------------------------------------------------------------------------------'
+ */
+ [_LOWER] = LAYOUT(
+ _______, _______, _______, _______, _______, _______, RGB_TOG, _______, _______, _______, _______, _______, _______, _______, _______,
+ _______, _______, _______, _______, _______, _______, RGB_VAI, _______, _______, _______, _______, _______, _______, _______, _______,
+ _______, _______, _______, _______, _______, _______, RGB_HUI, _______, _______, _______, _______, _______, _______, _______, _______,
+ _______, _______, _______, _______, _______, _______, RGB_SAI, _______, _______, _______, _______, _______, _______, _______, _______,
+ _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______
+ ),
+ /* ADJUST
+ * ,--------------------------------------------------------------------------------------------------------------------------------------.
+ * | | | | | | | | | | | | | | | |
+ * |--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------|
+ * | | | | | | | | | | | | | | | |
+ * |--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------|
+ * | | | | | | | | | | | | | | | |
+ * |--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------|
+ * | | | | | | | | | | | | | | | |
+ * |--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------+--------|
+ * | | | | | | | | | | | | | | | |
+ * `--------------------------------------------------------------------------------------------------------------------------------------'
+ */
+ [_ADJUST] = LAYOUT(
+ _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
+ _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
+ _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
+ _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
+ _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______
+ )
+};
diff --git a/keyboards/halfcliff/keymaps/via/readme.md b/keyboards/halfcliff/keymaps/via/readme.md
new file mode 100644
index 00000000000..2924c68c24a
--- /dev/null
+++ b/keyboards/halfcliff/keymaps/via/readme.md
@@ -0,0 +1 @@
+# The default keymap for halfcliff
diff --git a/keyboards/halfcliff/keymaps/via/rules.mk b/keyboards/halfcliff/keymaps/via/rules.mk
new file mode 100644
index 00000000000..1e5b99807cb
--- /dev/null
+++ b/keyboards/halfcliff/keymaps/via/rules.mk
@@ -0,0 +1 @@
+VIA_ENABLE = yes
diff --git a/keyboards/halfcliff/matrix.c b/keyboards/halfcliff/matrix.c
new file mode 100644
index 00000000000..556b75ca590
--- /dev/null
+++ b/keyboards/halfcliff/matrix.c
@@ -0,0 +1,277 @@
+/*
+Copyright 2012 Jun Wako
+
+This program is free software: you can redistribute it and/or modify
+it under the terms of the GNU General Public License as published by
+the Free Software Foundation, either version 2 of the License, or
+(at your option) any later version.
+
+This program is distributed in the hope that it will be useful,
+but WITHOUT ANY WARRANTY; without even the implied warranty of
+MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
+GNU General Public License for more details.
+
+You should have received a copy of the GNU General Public License
+along with this program. If not, see .
+*/
+#include
+#include
+#include "util.h"
+#include "matrix.h"
+#include "debounce.h"
+#include "quantum.h"
+#include "split_util.h"
+#include "config.h"
+#include "transport.h"
+
+#define ERROR_DISCONNECT_COUNT 5
+
+#define ROWS_PER_HAND (MATRIX_ROWS / 2)
+
+static pin_t row_pins[MATRIX_ROWS] = MATRIX_ROW_PINS;
+static pin_t col_pins[MATRIX_COLS] = MATRIX_COL_PINS;
+
+/* matrix state(1:on, 0:off) */
+static matrix_row_t raw_matrix[MATRIX_ROWS]; // raw values
+static matrix_row_t matrix[MATRIX_ROWS]; // debounced values
+
+// row offsets for each hand
+uint8_t thisHand, thatHand;
+
+// user-defined overridable functions
+__attribute__((weak)) void matrix_init_kb(void) { matrix_init_user(); }
+
+__attribute__((weak)) void matrix_scan_kb(void) { matrix_scan_user(); }
+
+__attribute__((weak)) void matrix_init_user(void) {}
+
+__attribute__((weak)) void matrix_scan_user(void) {}
+
+__attribute__((weak)) void matrix_slave_scan_user(void) {}
+
+matrix_row_t matrix_get_row(uint8_t row) { return matrix[row]; }
+
+void matrix_print(void) {}
+
+static inline void setPinOutput_writeLow(pin_t pin) {
+ ATOMIC_BLOCK_FORCEON {
+ setPinOutput(pin);
+ writePinLow(pin);
+ }
+}
+
+static inline void setPinInputHigh_atomic(pin_t pin) {
+ ATOMIC_BLOCK_FORCEON { setPinInputHigh(pin); }
+}
+
+// matrix code
+static void select_row(uint8_t row) { setPinOutput_writeLow(row_pins[row]); }
+
+static void unselect_row(uint8_t row) { setPinInputHigh_atomic(row_pins[row]); }
+
+static void unselect_rows(void) {
+ for (uint8_t x = 0; x < ROWS_PER_HAND; x++) {
+ setPinInputHigh_atomic(row_pins[x]);
+ }
+}
+
+static bool read_cols_on_row(matrix_row_t current_matrix[], uint8_t current_row) {
+ // Start with a clear matrix row
+ matrix_row_t current_row_value = 0;
+
+ // Select row
+ select_row(current_row);
+ wait_us(30);
+
+ // For each col...
+ for (uint8_t col_index = 0; col_index < MATRIX_COLS; col_index++) {
+ // Select the col pin to read (active low)
+ uint8_t pin_state = readPin(col_pins[col_index]);
+
+ // Populate the matrix row with the state of the col pin
+ current_row_value |= pin_state ? 0 : (MATRIX_ROW_SHIFTER << col_index);
+ }
+
+ // Unselect row
+ unselect_row(current_row);
+ if (current_row + 1 < MATRIX_ROWS) {
+ wait_us(30); // wait for row signal to go HIGH
+ }
+
+ // If the row has changed, store the row and return the changed flag.
+ if (current_matrix[current_row] != current_row_value) {
+ current_matrix[current_row] = current_row_value;
+ return true;
+ }
+ return false;
+}
+
+static void select_col(uint8_t col) { setPinOutput_writeLow(col_pins[col]); }
+
+static void unselect_col(uint8_t col) { setPinInputHigh_atomic(col_pins[col]); }
+
+static void unselect_cols(void) {
+ for (uint8_t x = 0; x < MATRIX_COLS; x++) {
+ setPinInputHigh_atomic(col_pins[x]);
+ }
+}
+
+static void init_pins(void) {
+ unselect_rows();
+ for (uint8_t x = 0; x < MATRIX_COLS; x++) {
+ setPinInputHigh_atomic(col_pins[x]);
+ }
+ unselect_cols();
+ for (uint8_t x = 0; x < ROWS_PER_HAND; x++) {
+ setPinInputHigh_atomic(row_pins[x]);
+ }
+}
+
+static bool read_rows_on_col(matrix_row_t current_matrix[], uint8_t current_col) {
+ bool matrix_changed = false;
+
+ // Select col
+ select_col(current_col);
+ wait_us(30);
+
+ // For each row...
+ for (uint8_t row_index = 0; row_index < ROWS_PER_HAND; row_index++) {
+ // Store last value of row prior to reading
+ matrix_row_t last_row_value = current_matrix[row_index];
+ matrix_row_t current_row_value = last_row_value;
+
+ // Check row pin state
+ if (readPin(row_pins[row_index]) == 0) {
+ // Pin LO, set col bit
+ current_row_value |= (MATRIX_ROW_SHIFTER << current_col);
+ } else {
+ // Pin HI, clear col bit
+ current_row_value &= ~(MATRIX_ROW_SHIFTER << current_col);
+ }
+
+ // Determine if the matrix changed state
+ if ((last_row_value != current_row_value)) {
+ matrix_changed |= true;
+ current_matrix[row_index] = current_row_value;
+ }
+ }
+
+ // Unselect col
+ unselect_col(current_col);
+ if (current_col + 1 < MATRIX_COLS) {
+ wait_us(30); // wait for col signal to go HIGH
+ }
+
+ return matrix_changed;
+}
+
+void matrix_init(void) {
+ split_pre_init();
+
+ // Set pinout for right half if pinout for that half is defined
+ if (!isLeftHand) {
+#ifdef DIRECT_PINS_RIGHT
+ const pin_t direct_pins_right[MATRIX_ROWS][MATRIX_COLS] = DIRECT_PINS_RIGHT;
+ for (uint8_t i = 0; i < MATRIX_ROWS; i++) {
+ for (uint8_t j = 0; j < MATRIX_COLS; j++) {
+ direct_pins[i][j] = direct_pins_right[i][j];
+ }
+ }
+#endif
+#ifdef MATRIX_ROW_PINS_RIGHT
+ const pin_t row_pins_right[MATRIX_ROWS] = MATRIX_ROW_PINS_RIGHT;
+ for (uint8_t i = 0; i < MATRIX_ROWS; i++) {
+ row_pins[i] = row_pins_right[i];
+ }
+#endif
+#ifdef MATRIX_COL_PINS_RIGHT
+ const pin_t col_pins_right[MATRIX_COLS] = MATRIX_COL_PINS_RIGHT;
+ for (uint8_t i = 0; i < MATRIX_COLS; i++) {
+ col_pins[i] = col_pins_right[i];
+ }
+#endif
+ }
+
+ thisHand = isLeftHand ? 0 : (ROWS_PER_HAND);
+ thatHand = ROWS_PER_HAND - thisHand;
+
+ // initialize key pins
+ init_pins();
+
+ // initialize matrix state: all keys off
+ for (uint8_t i = 0; i < MATRIX_ROWS; i++) {
+ raw_matrix[i] = 0;
+ matrix[i] = 0;
+ }
+
+ debounce_init(ROWS_PER_HAND);
+
+ matrix_init_quantum();
+
+ split_post_init();
+}
+
+bool matrix_post_scan(void) {
+ bool changed = false;
+ if (is_keyboard_master()) {
+ static uint8_t error_count;
+
+ matrix_row_t slave_matrix[ROWS_PER_HAND] = {0};
+ if (!transport_master(matrix + thisHand, slave_matrix)) {
+ error_count++;
+
+ if (error_count > ERROR_DISCONNECT_COUNT) {
+ // reset other half if disconnected
+ for (int i = 0; i < ROWS_PER_HAND; ++i) {
+ matrix[thatHand + i] = 0;
+ slave_matrix[i] = 0;
+ }
+
+ changed = true;
+ }
+ } else {
+ error_count = 0;
+
+ for (int i = 0; i < ROWS_PER_HAND; ++i) {
+ if (matrix[thatHand + i] != slave_matrix[i]) {
+ matrix[thatHand + i] = slave_matrix[i];
+ changed = true;
+ }
+ }
+ }
+
+ matrix_scan_quantum();
+ } else {
+ transport_slave(matrix + thatHand, matrix + thisHand);
+
+ matrix_slave_scan_user();
+ }
+
+ return changed;
+}
+
+uint8_t matrix_scan(void) {
+ bool local_changed = false;
+ static matrix_row_t temp_raw_matrix[MATRIX_ROWS]; // temp raw values
+
+ // Set row, read cols
+ for (uint8_t current_row = 0; current_row < ROWS_PER_HAND/2; current_row++) {
+ local_changed |= read_cols_on_row(raw_matrix, current_row);
+ }
+
+ // Set col, read rows
+ for (uint8_t current_col = 0; current_col < MATRIX_COLS; current_col++) {
+ local_changed |= read_rows_on_col(temp_raw_matrix, current_col);
+ //Updated key matrix on lines 6-10 (or lines 16-20)
+ if(local_changed) {
+ for (uint8_t i = ROWS_PER_HAND/2; i < ROWS_PER_HAND; i++) {
+ raw_matrix[i] = temp_raw_matrix[i];
+ }
+ }
+ }
+
+ debounce(raw_matrix, matrix + thisHand, ROWS_PER_HAND, local_changed);
+
+ bool remote_changed = matrix_post_scan();
+ return (uint8_t)(local_changed || remote_changed);
+}
diff --git a/keyboards/halfcliff/readme.md b/keyboards/halfcliff/readme.md
new file mode 100644
index 00000000000..37e8d85c20f
--- /dev/null
+++ b/keyboards/halfcliff/readme.md
@@ -0,0 +1,19 @@
+# halfcliff
+
+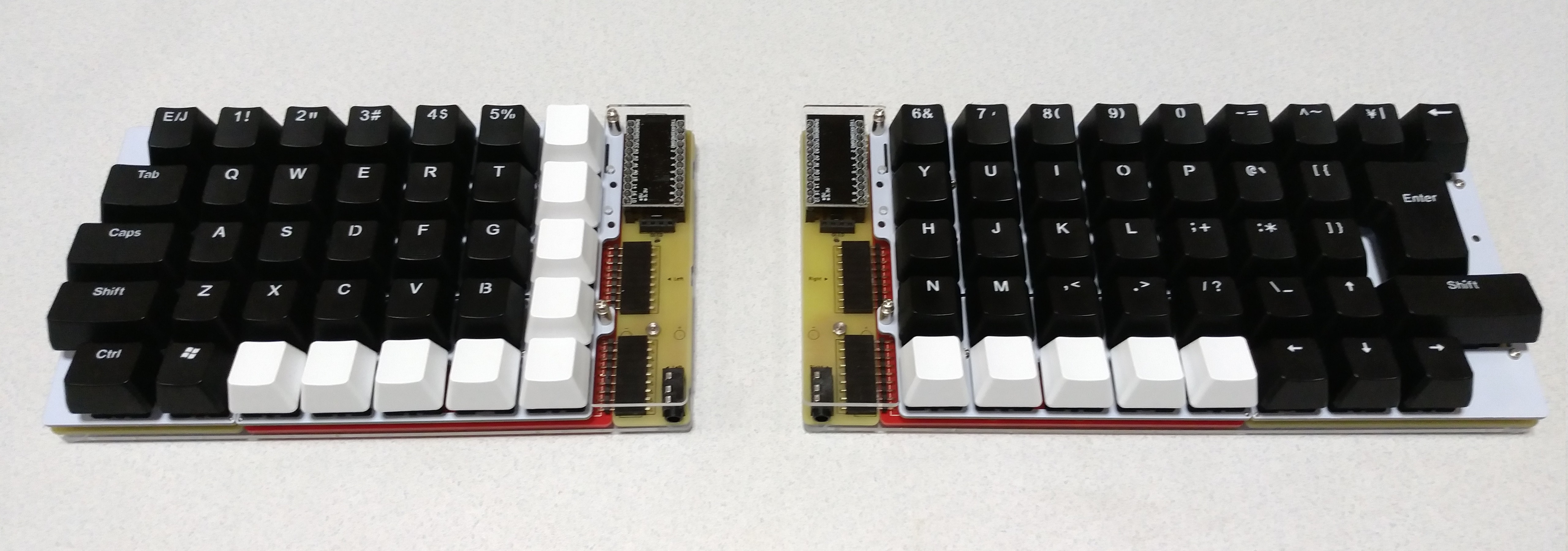
+
+Halfcliff keyboard is a split keyboard using a single-sided board.
+
+* Keyboard Maintainer: [n2](https://github.com/N2-Sumikko)
+* Hardware Supported: halfcliff PCB, Pro Micro
+* Hardware Availability: [PCB & Plate Data](https://github.com/N2-Sumikko/HalfCliff.git)
+
+Make example for this keyboard (after setting up your build environment):
+
+ make halfcliff:default
+
+Flashing example for this keyboard:
+
+ make halfcliff:default:flash
+
+See the [build environment setup](https://docs.qmk.fm/#/getting_started_build_tools) and the [make instructions](https://docs.qmk.fm/#/getting_started_make_guide) for more information. Brand new to QMK? Start with our [Complete Newbs Guide](https://docs.qmk.fm/#/newbs).
diff --git a/keyboards/halfcliff/rules.mk b/keyboards/halfcliff/rules.mk
new file mode 100644
index 00000000000..f8883f6a477
--- /dev/null
+++ b/keyboards/halfcliff/rules.mk
@@ -0,0 +1,29 @@
+# MCU name
+MCU = atmega32u4
+
+# Bootloader selection
+BOOTLOADER = caterina
+
+# Build Options
+# change yes to no to disable
+#
+BOOTMAGIC_ENABLE = no # Virtual DIP switch configuration
+MOUSEKEY_ENABLE = no # Mouse keys
+EXTRAKEY_ENABLE = no # Audio control and System control
+CONSOLE_ENABLE = no # Console for debug
+COMMAND_ENABLE = no # Commands for debug and configuration
+# Do not enable SLEEP_LED_ENABLE. it uses the same timer as BACKLIGHT_ENABLE
+SLEEP_LED_ENABLE = no # Breathing sleep LED during USB suspend
+# if this doesn't work, see here: https://github.com/tmk/tmk_keyboard/wiki/FAQ#nkro-doesnt-work
+NKRO_ENABLE = no # USB Nkey Rollover
+BACKLIGHT_ENABLE = no # Enable keyboard backlight functionality
+RGBLIGHT_ENABLE = no # Enable keyboard RGB underglow
+BLUETOOTH_ENABLE = no # Enable Bluetooth
+AUDIO_ENABLE = no # Audio output
+SPLIT_KEYBOARD = yes
+ENCODER_ENABLE = no
+POINTING_DEVICE_ENABLE = no
+CUSTOM_MATRIX = yes
+OLED_DRIVER_ENABLE = no
+
+SRC += matrix.c